Error Handling in PowerShell
In PowerShell, you can use use the try, catch, and finally
blocks to handle terminating errors and stops a statement from running.
- A
try
block is used to define a section of a script in which you want PowerShell to monitor for errors.
- A
catch
block is used to handle the error.
- A
finally
block can be used to free any resources that are no longer needed by your script.
Try, Catch Finally Syntax
try {
# your code
}
catch {
Write-Host "An error occurred:"
# to get error details, use one of the below
Write-Host $_
Write-Host $Error
Write-Host $_.Exception
}
finally{
# free any resources that are no longer needed
}
Output
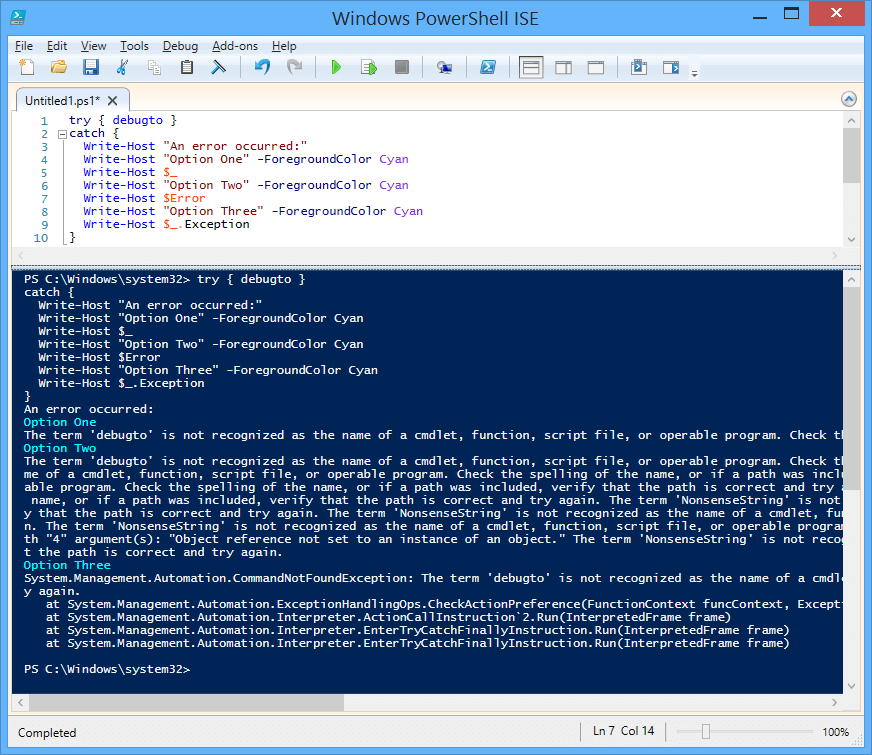
When an error occurs within the try block, the error is first saved to the $Error
automatic variable, and If the error cannot be handled, the error is written to the error stream.
Solving: Try, Catch is not working in PowerShell
In your case, if your code is not getting caught, try to use -ErrorAction stop
as below
try {
# your code -ErrorAction stop
}
catch {
Write-Host "An error occurred:"
Write-Host $Error
}