Validate Email in ASP.NET
You can easily validate an Email Address without using any kind of code using Regular Expressions control in ASP.NET as the following:
- In your Project, Add a Regular Expression Validator from the toolbox.
- Click on your
Regular Expression Validator
control, then click on "F4
" to open its properties.
- In the properties, click on Validation Expression, then select
Internet E-Mail Address
.
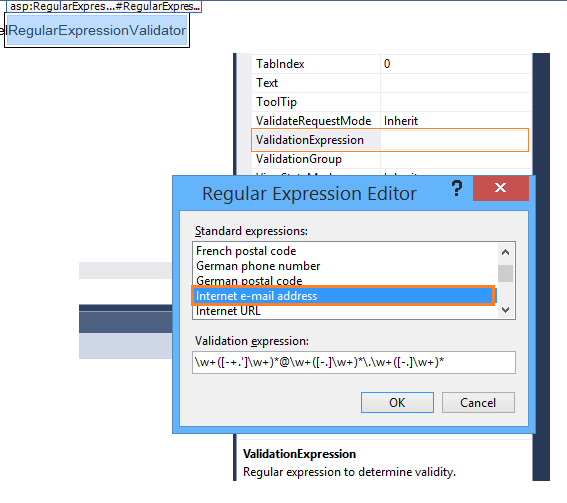
- After that, make sure you have set the following properties:
- ControlToValidate
- ValidationGroup
- ErrorMessage
Output
<asp:TextBox ID="txt_email" runat="server"/>
<asp:RegularExpressionValidator ID="EmailValidator" runat="server" ValidationExpression="\w+([-+.]\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*" ControlToValidate="txt_email" ErrorMessage="Invalid Email Address" ValidationGroup="Group1"></asp:RegularExpressionValidator>
Besides the above method, you can also use the below function to validate Email Address using C# as below
public bool EmailAddressValidator(string email)
{
if (new EmailAddressAttribute().IsValid(email) && !string.IsNullOrEmpty(email))
return true;
else
return false;
}