Which data type should I use in SQL to store Image in Base64?
Since the API returns a base64 string, you should be able to insert the image with dataType varchar(MAX)
and the image will be stored without any issues.
The max size of 'varchar(MAX)' is 2GB.

Convert Image to Base64 using C#.
And in your code, you have to convert your image field to base64 as below:
byte[] imageArray = System.IO.File.ReadAllBytes(@"image file path");
string base64Image= Convert.ToBase64String(imageArray);
Get an image in base64 format from SQL server to image tag in HTML using C#.
To retrieve or get an image in base64 format from the SQL server, you have to do the following:
<img src="data:image/png;base64, YourValue">
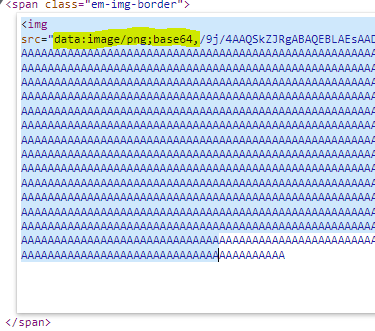
So in our case, we can use the below code to bind your image in Base64 to the <img>
tag in HTML and bind the field name as below
<img src="data:image/png;base64, <%# Eval("FieldName") %>" />
In case the image is returned as bytes and you would like to use it as base 64, you can use the below code
<img src="data:image/png;base64, <%# Convert.Base64String(byte[] Eval("FieldName")) %>" />