How to Prevent input text from accepting any characters other than Arabic and DOT(.) using regular expressions?
To Prevent input text from accepting any characters other than Arabic and DOT(.) using regular expressions in MVC, you should follow the below steps:
- Navigate to the
_Layout.cshtml
file.
- Add the
<script></script>
tags below.
MVC Text Box allow only Arabic characters in FrontEnd
- Open
_Layout.cshtml
file
Add the <script></script>
tags below
$(".arabicWithDot").on("input", function () {
var inputValue = $(this).val();
var validChars = /^[\u0600-\u06FF\s.]+$/;
if (!validChars.test(inputValue)) {
// Remove invalid characters
$(this).val(inputValue.replace(/[^\u0600-\u06FF\s.]+/g, ''));
}
});
Add arabicWithDot class to any input type to allow only Arabic character and DOT (.)
<div class="mb-3 col-xs-12 col-sm-6 col-md-6 col-lg-6">
@Html.Label("Resource.Label.JobTitle", new { @class = " form-label", @for = "Position" })
<span style="color: red;">*</span>
@Html.TextBoxFor(m => m.Position, new { @class = "form-control arabicWithDot", @maxlength = "250" })
@Html.ValidationMessageFor(Model => Model.Position, null, new { @class = "text-danger" })
</div>
MVC Text Box allows only Arabic characters using BackEnd
- Open your
ViewModel
class file
- Add the
public string Position { get; set; }
into ViewModel Class
Add RegularExpression above property allowing only Arabic characters and DOT (.)
[RegularExpression(@"^[\u0600-\u06FF\s.]+$", ErrorMessage ="Arabic and Dot Only")]
public string Position { get; set; }
Result:
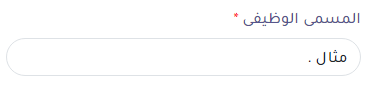
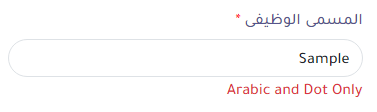