In this article, you will learn the fundamentals of the Python programming language, along with programming best practices.
- Also, you will learn to represent and store data using Python data types and variables, and use conditionals and loops to control the flow of your programs.
- In addition, you will be familiar to harness the power of complex data structures like lists, tuples, and dictionaries to store collections of related data.
- Moreover, you will know the differences between python and c++.
- Lastly, you will be able to define and document your own custom functions, write scripts, and handle errors.
What is Python?
Python is a popular programming language in web development and data science. It was created by Guido van Rossum, and released in 1991.
- It has a simple syntax that allows developers to write programs with fewer lines than some other programming languages.
- It works on different platforms (Windows, Mac, Linux, Raspberry Pi, etc). As every Programming Language has an extension, it has an extension called (. py). All Python files are stored in this extension.
- It is used by New York Stock Exchange, Youtube, and Google search engines.
- Lastly, The most popular framework of Python are flask and django.
Python data types
Following are the data type of Python:
- Integer
- Float
- Boolean
- None
What is Integer?
The value is represented by int class. It contains positive or negative whole numbers without decimal and there is no limit to how long an integer value can be.
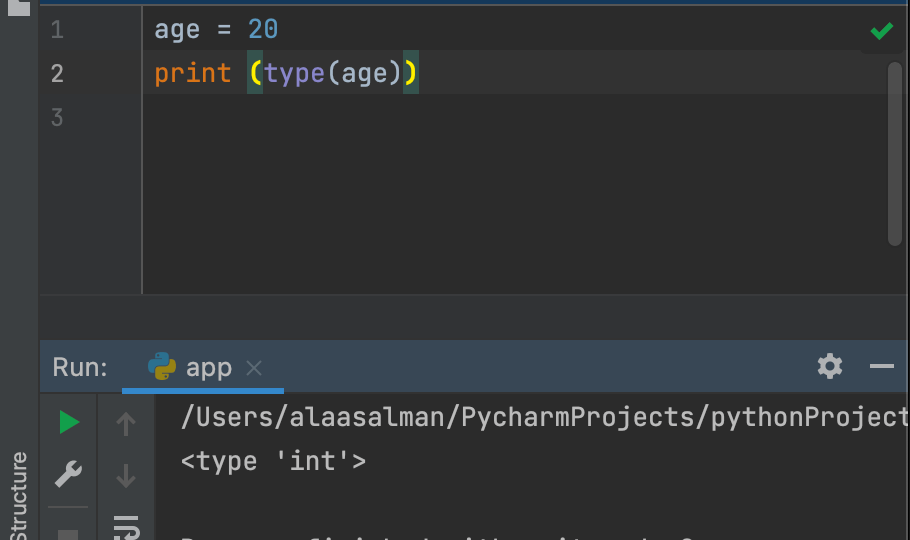
age = 20
print (type(age))
What is Float?
The value is represented by float class. It is a real number with floating point representation. It is specified by a decimal point.
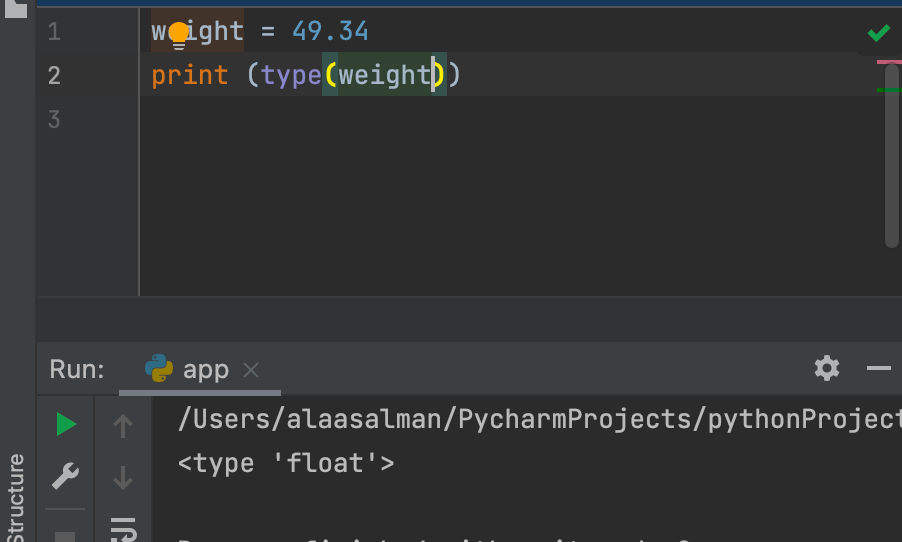
weight = 49.34
print (type(weight))
What is Boolean?
Data type with one of the two built-in values, True or False.
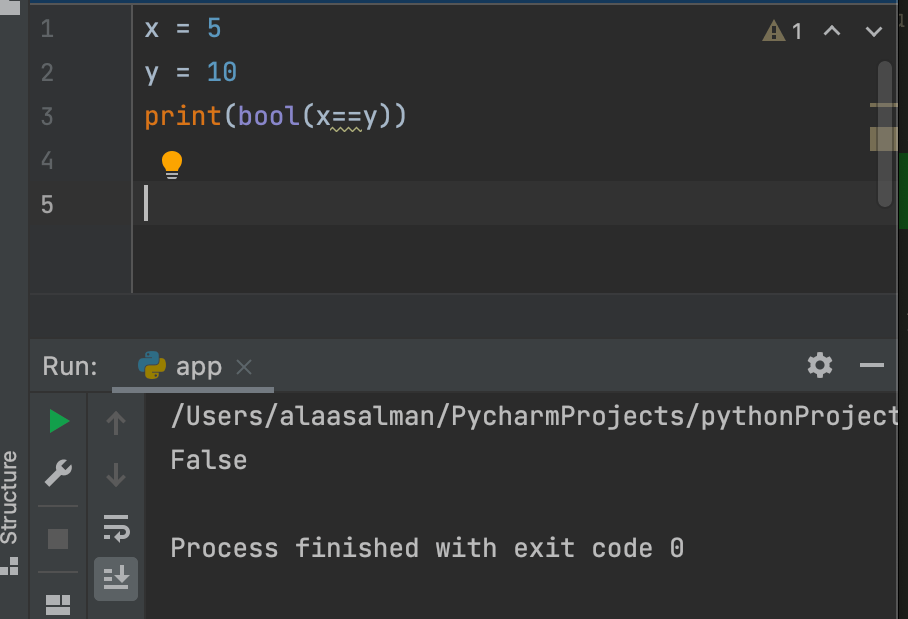
x = 5
y = 10
print (bool(x==y))
What is None?
The none is used to define a null value, or no value at all.
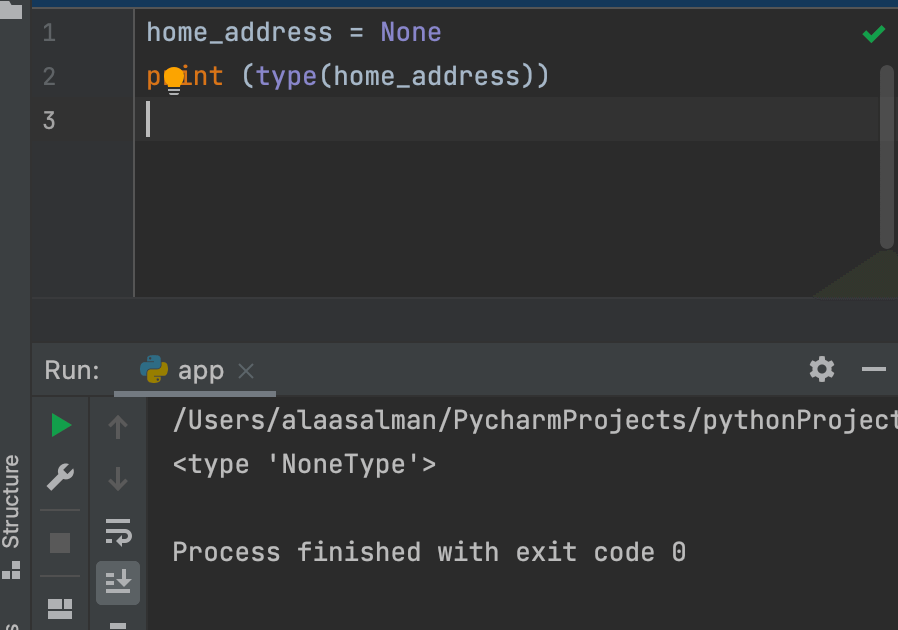
home_address = None
print (type(home_address))
What is Sequence Type?
Sequence is the ordered collection of similar or different data types. Sequences allows to store multiple values in an organized and efficient fashion
Following are the Sequence Type of Python:
What is String?
Strings are a special type of sequence that can only store characters.
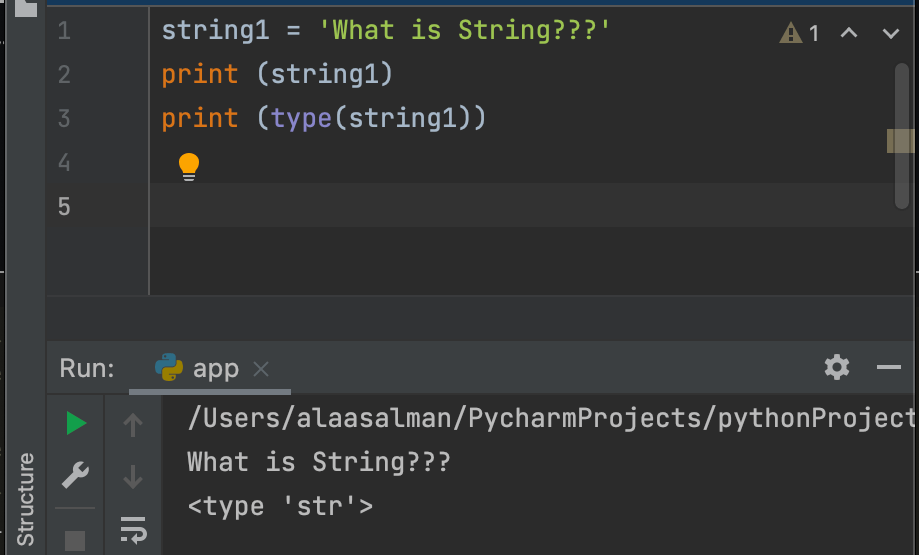
string1 = 'What us String???'
print (string1)
print (type(string1))
What is list?
The list allows us to work with multiple elements at once and it can be any number of items and they may be of different types (integer, float, string, etc.). Also, lists can be changed. Elements can be reassigned or removed, and new elements can be inserted.
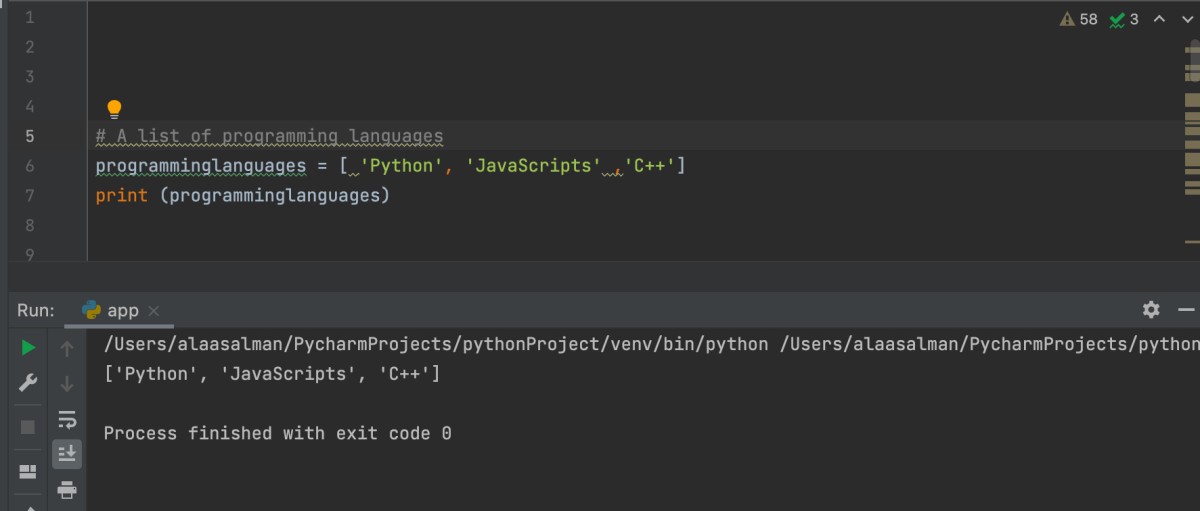
# A list of programming languages
programminglanguages = [ 'Python', 'JavaScripts' ,'C++']
print (programminglanguages)
How to change the elements?
by the number of the index.

# A list of programming languages
programminglanguages = [ 'Python', 'JavaScripts' ,'C++']
programminglanguages [2] = 'PHP'
print (programminglanguages)
Note: the hash character use to write comments in computer programs that are ignored by compilers and interpreters. Using comments in programs can make code more readable as it provides explanation about what each part of a program is doing.
How to insert new element?
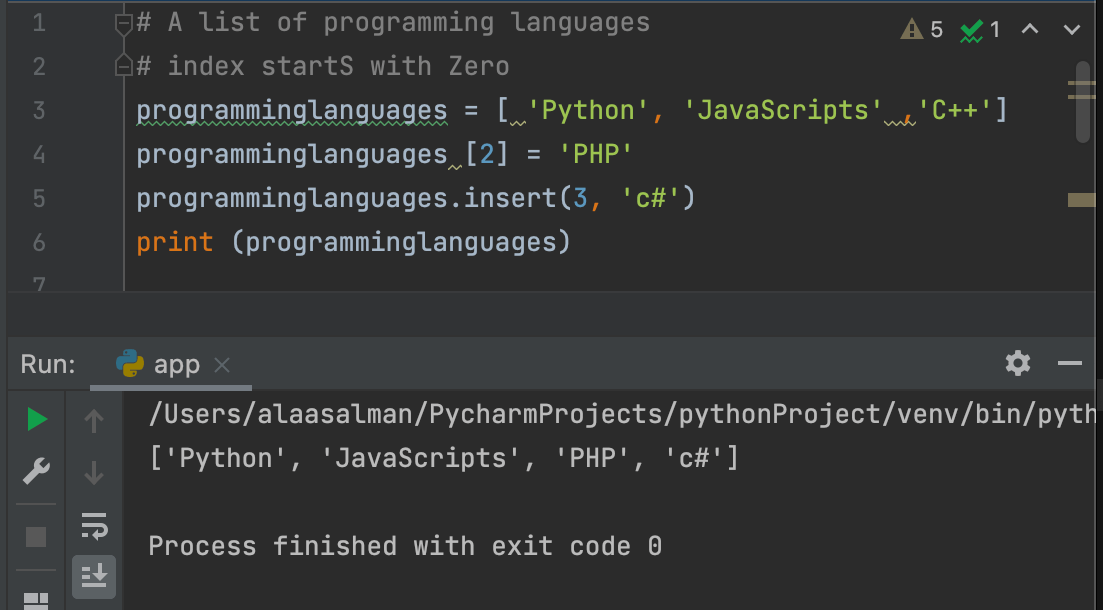
# A list of programming languages
# index starts from Zero
programminglanguages = [ 'Python', 'JavaScripts' ,'C++']
programminglanguages [2] = 'PHP'
programminglanguages.insert(3, 'c#')
print (programminglanguages)
What is Tuple?
Tuple are like lists but they can't be changed.
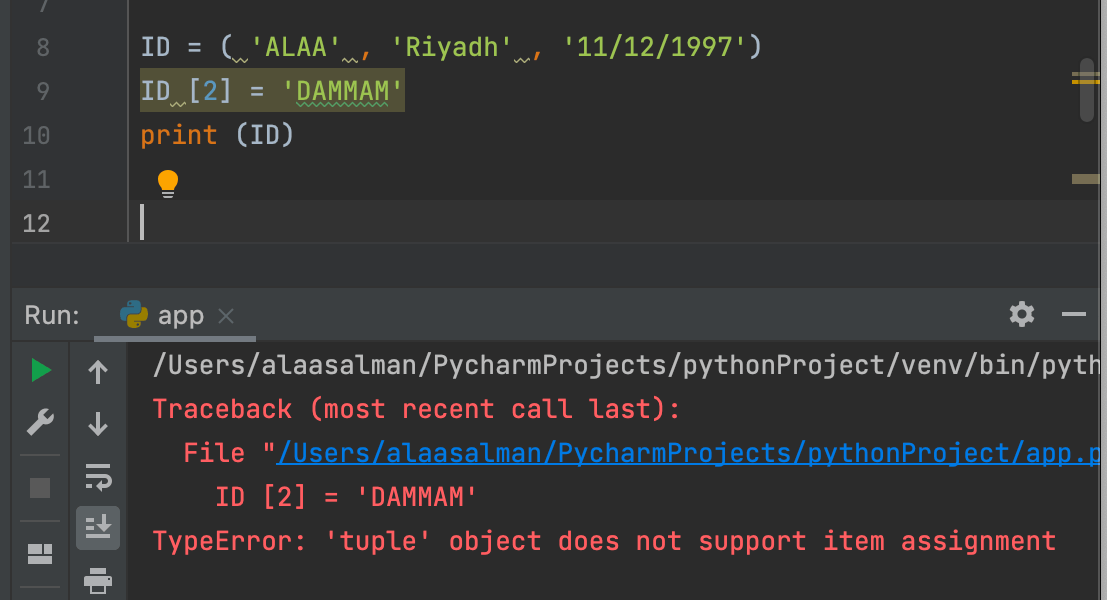
ID = ('ALAA' , 'Riyadh' , '11/12/1997')
ID [2] = 'DAMMAM'
print (ID)
What is dictionary?
The dictionary is made up a set of keys and values where there is a mapping between a given key and its corresponding value. The main difference is that elements in dictionaries are accessed via keys and not via their position. Hence, we can get a value from the dictionary by its key very quickly Unlike other types.
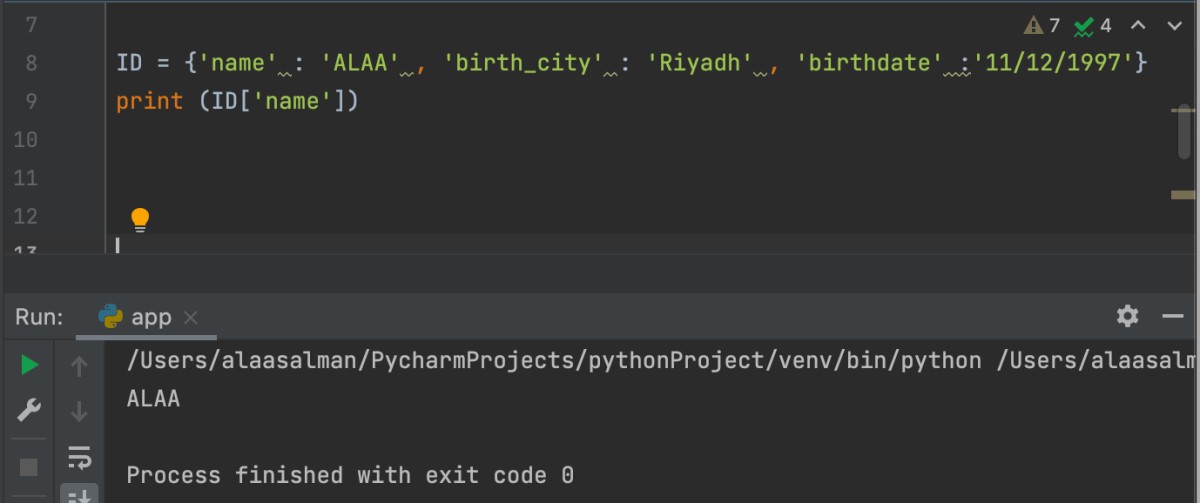
ID = {'name' : 'ALAA' , 'birth_city' : 'Riyadh' , 'birthdate' :'11/12/1997'}
print (ID['name'])
Logical Operators
Python has three Logical Boolean operators are
Operators
|
What it means
|
And
|
True if both are true
|
Or
|
True if at least one is true
|
Not
|
True only if it’s false
|
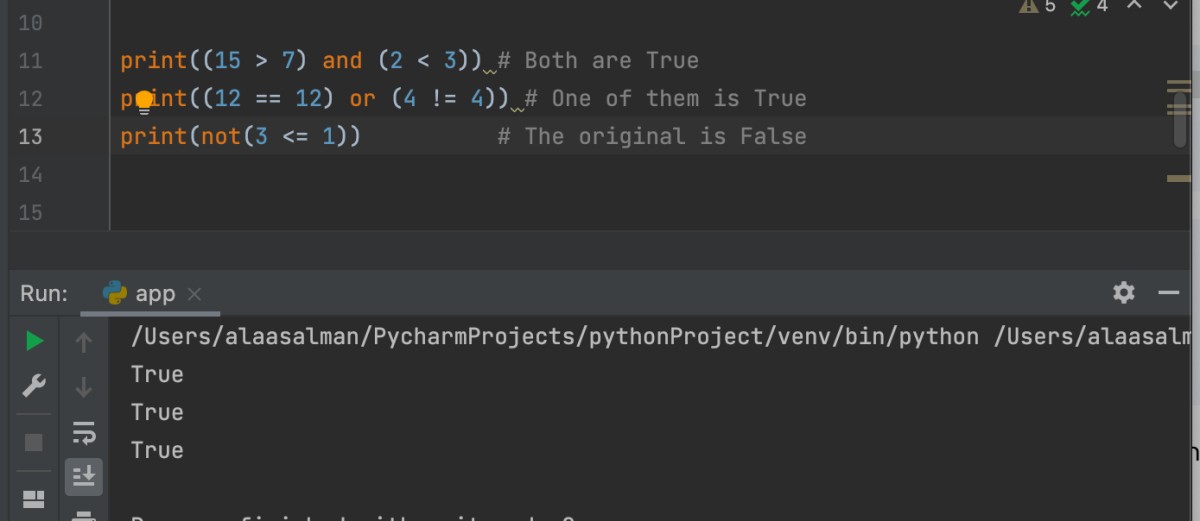
print((15 > 7) and (2 < 3)) # Both are True
print((12 == 12) or (4 != 4)) # One of them is True
print(not(3 <= 1)) # The original is False
IF, ELIF, and ELSE Statements
- IF Statement is used for decision-making operations.
- The ELIF keyword is used when the previous conditions were not true, then try this condition.
- The ELSE keyword catches anything which isn't caught by the preceding.
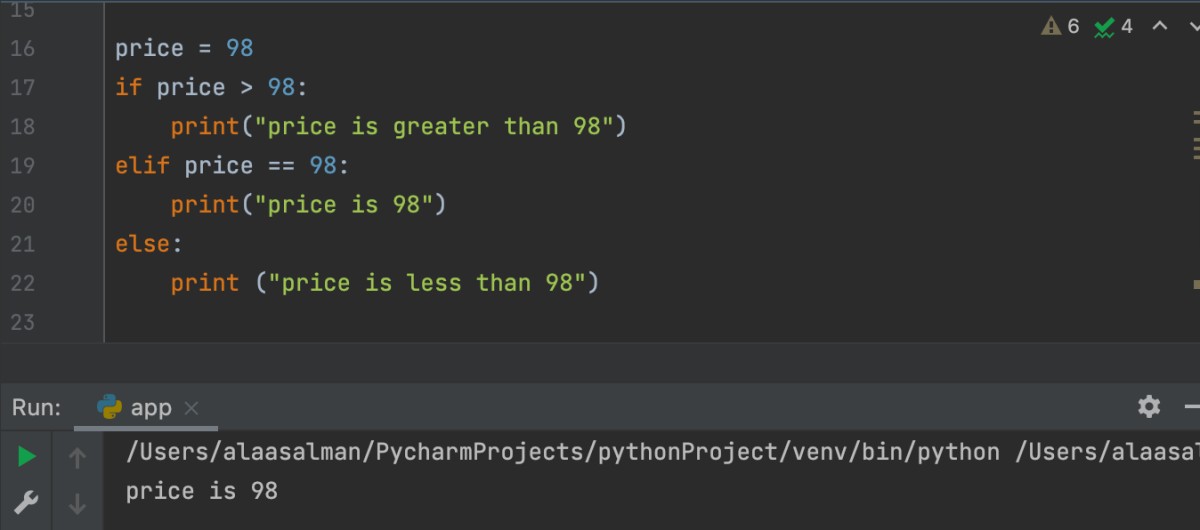
price = 98
if price > 98:
print("price is greater than 98")
elif price == 98:
print("price is 98")
else:
print ("price is less than 98")
Python Loops
What does Loop is used for?
It is used for repeating over a sequence.
Python has two primitive loop commands:
While Loops Example
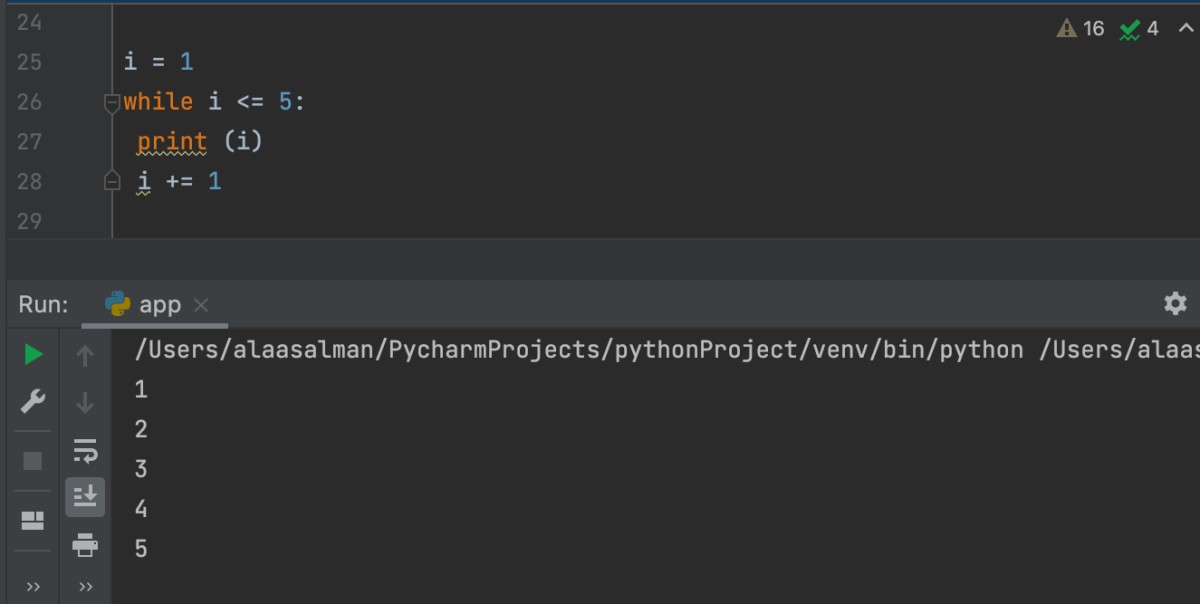
i = 1
while i <= 5:
print (i)
i += 1
For Loops Example
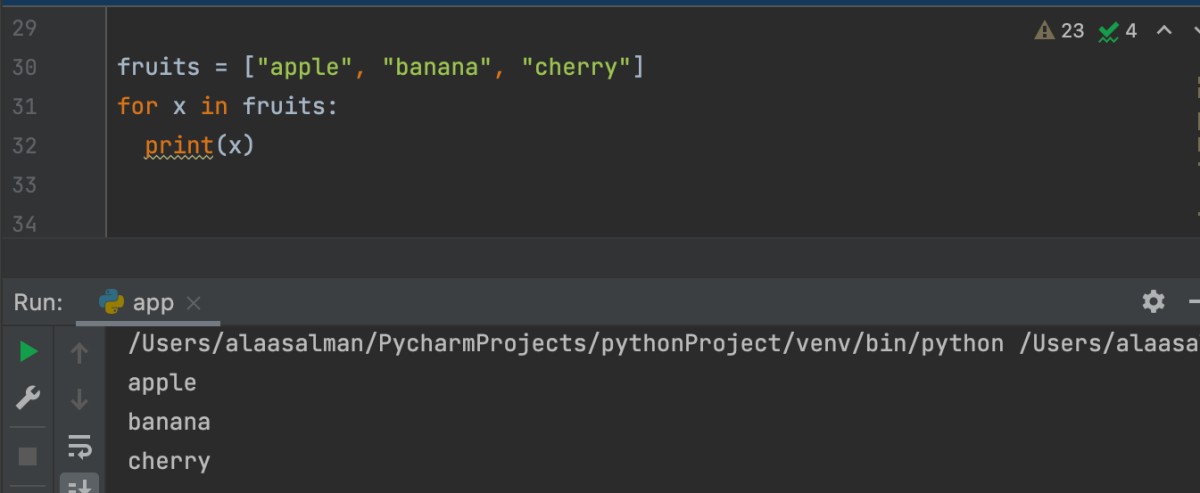
fruits = ["apple", "banana", "cherry"]
for x in fruits:
print(x)
Note: increment i, or the loop will continue forever.
Python Functions
What is Function?
- A function is a block of code which only runs when it is called.
- a function is defined using the def keyword
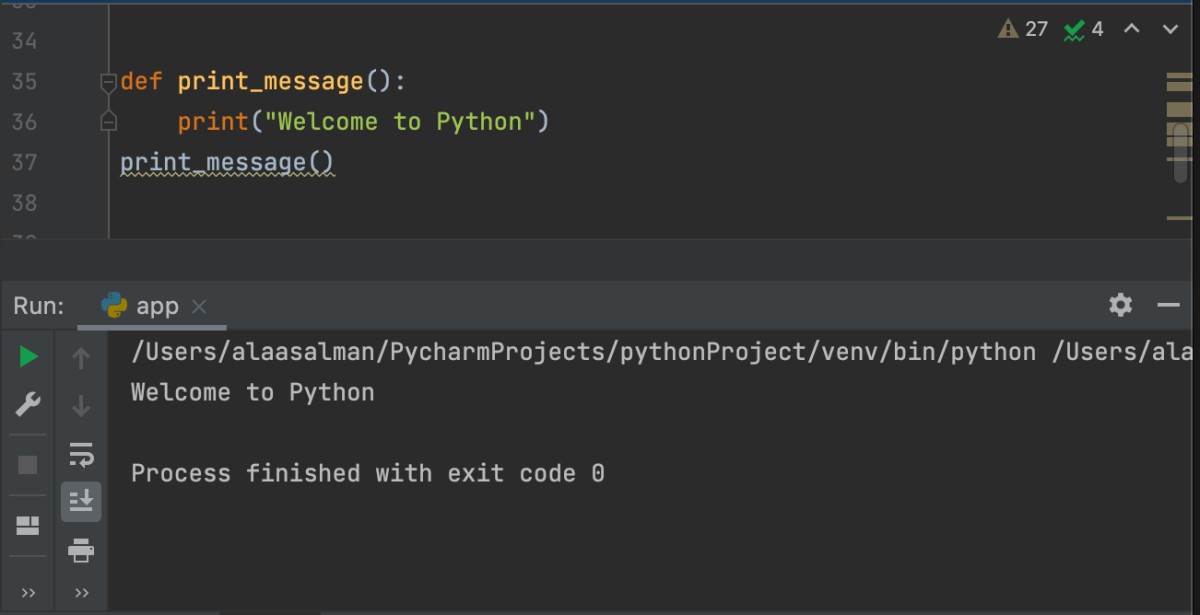
def print_message():
print("Welcome to Python")
print_message()
Python vs C++
C++ is a high-level, general-purpose programming language created by Bjarne Stroustrup as an extension of the C programming language. The language has expanded significantly over time, and modern C++ has object-oriented, generic, and functional features in addition to facilities for low-level memory manipulation.
In the below table we will list the main differences between structured programming and object-oriented programming
Parmeter
|
Python
|
C++
|
Code
|
less lines of code.
|
large lines of code.
|
Garbage Collection
|
supports garbage collection
|
doesn’t support garbage collection.
|
Syntax
|
easy to be remembered almost similar to human language.
|
A stiff learning curve as it has many of predefined syntaxes and structure
|
Compilation
|
uses interpreter
|
pre compiled.
|
Speed
|
slower since it uses interpreter
|
faster in speed as compared to python
|
Rapid Prototyping
|
possible due to the small size of the code.
|
not possible due to larger code size.
|
Efficiency
|
Easier to maintain, object-oriented and simpler to use
|
Less clean and manageable in comparison to python
|
Nature
|
dynamically typed.
|
statically typed.
|
Extension
|
saved with .py extension.
|
saved with .cpp extension.
|
Conclusion
Python is better for beginners in terms of its easy-to-read code and simple syntax. Additionally, it is a good option for web development (backend). It uses new lines to complete a command, as opposed to other programming languages which often use semicolons or parentheses. Also, it comes with a large standard library that has some handy codes and functions which we can use while writing code in Python. Lastly, it is an open-source programming language.
References: