In this article, you will learn the indexing vs slicing, raw string, list comprehension, 3 types of arguments,Arguments packing & unpacking, How to create constructor, What Are Getters And Setters and Access modifiers.
Indexing vs Slicing
What is Indexing?
It creates a reference to an element of an iterable (tuples, strings, list) by its particular position.
How does indexing work?
It searches for a given element in string, list and tuple.
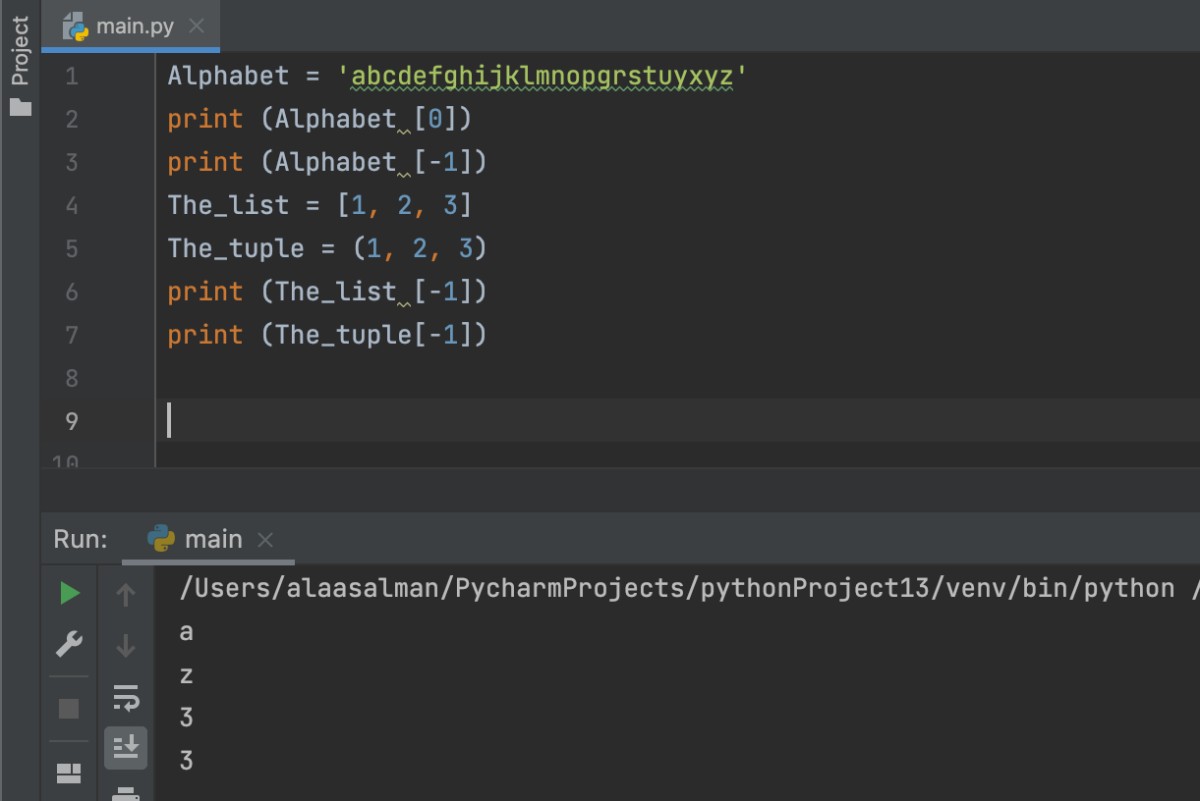
Alphabet = 'abcdefghijklmnopgrstuyxyz'
print (Alphabet [0])
print (Alphabet [-1])
The_list = [1, 2, 3]
The_tuple = (1, 2, 3)
print (The_list [-1])
print (The_tuple[-1])
What is Slicing?
Slicing is a subset of all elements from an iterable (tuples, strings, list) based on their indexes.
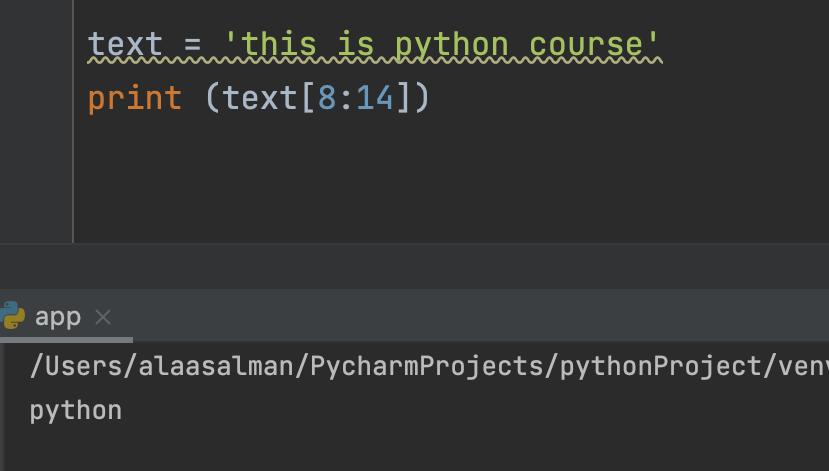
text = 'this is python course'
print (text[8:14])
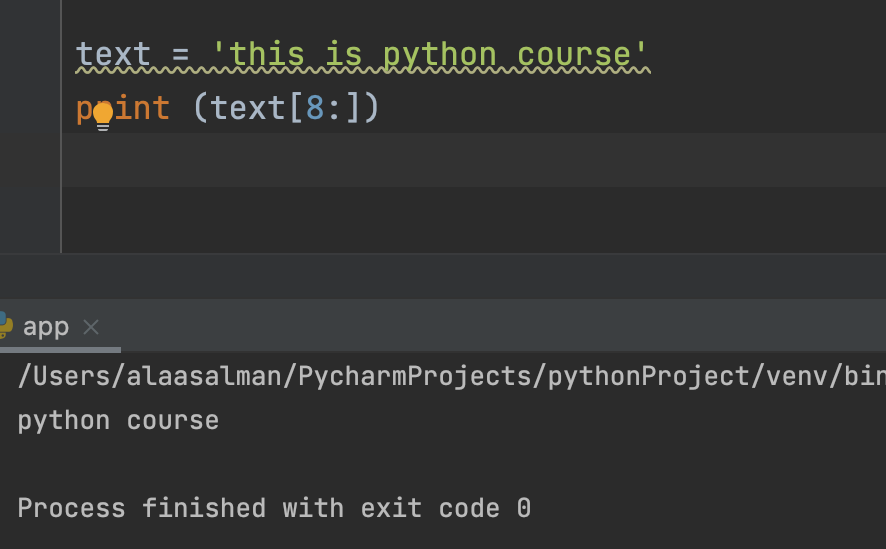
text = 'this is python course'
print (text[8:])
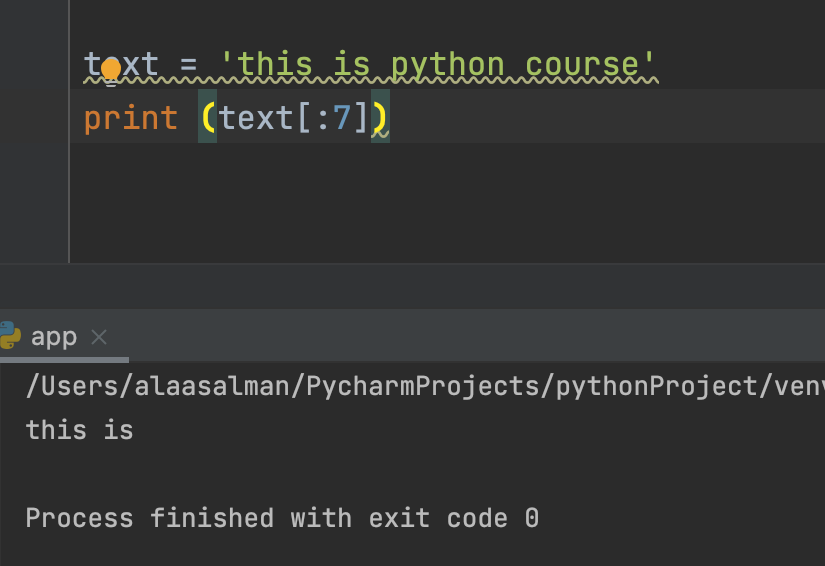
text = 'this is python course'
print (text[:7])
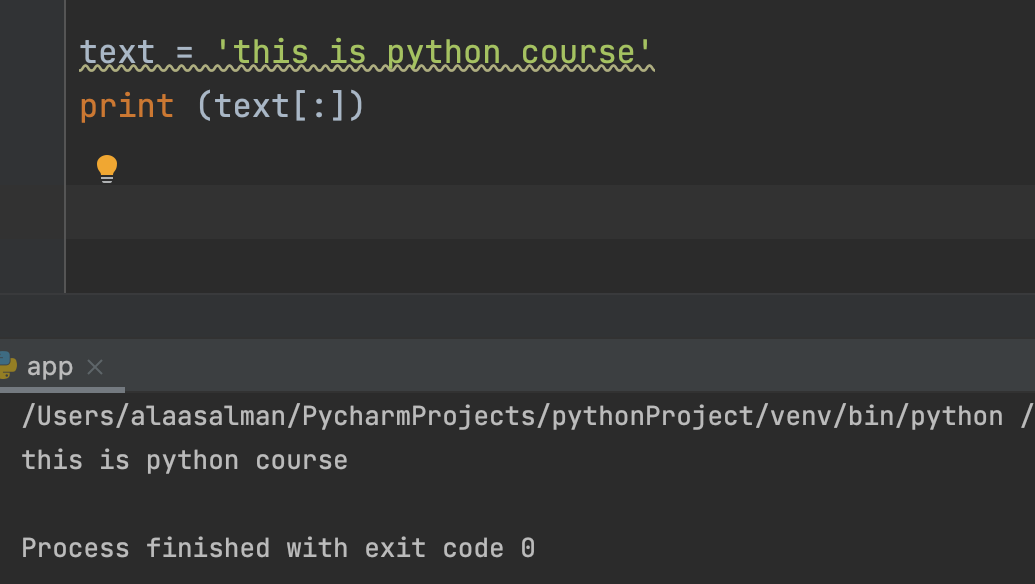
text = 'this is python course'
print (text[:])
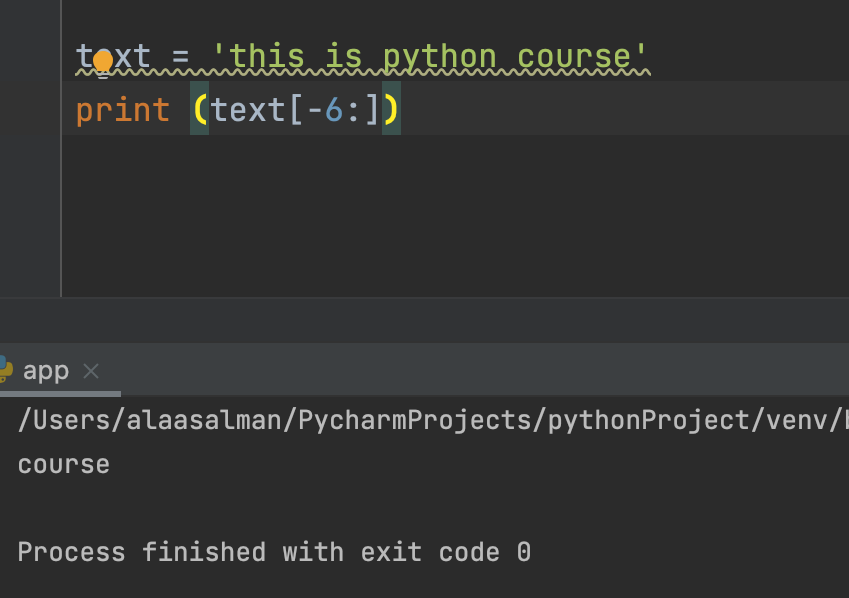
text = 'this is python course'
print (text[-6:])
-
How do you skip an element in a list?
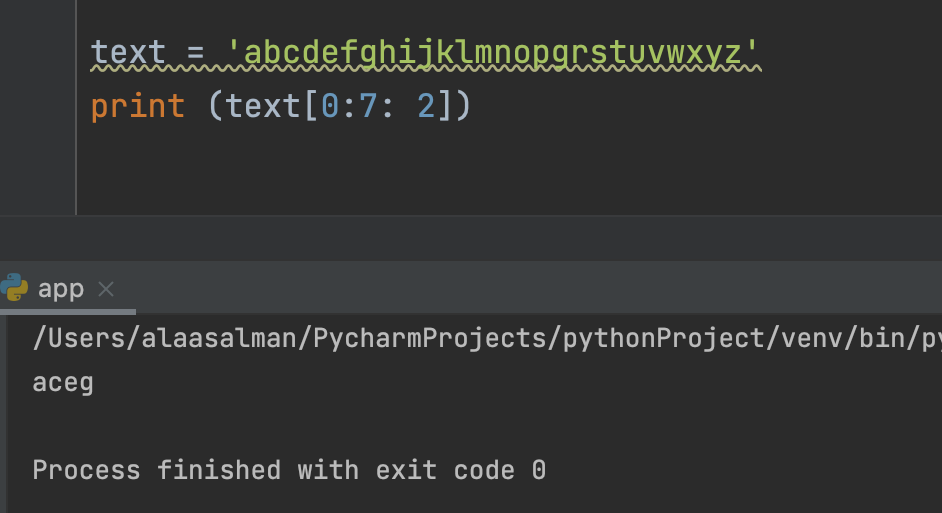
text = 'abcdefghijklmnopgrstuvwxyz'
print (text[0:7: 2])
-
How do you check if an element in a list ?
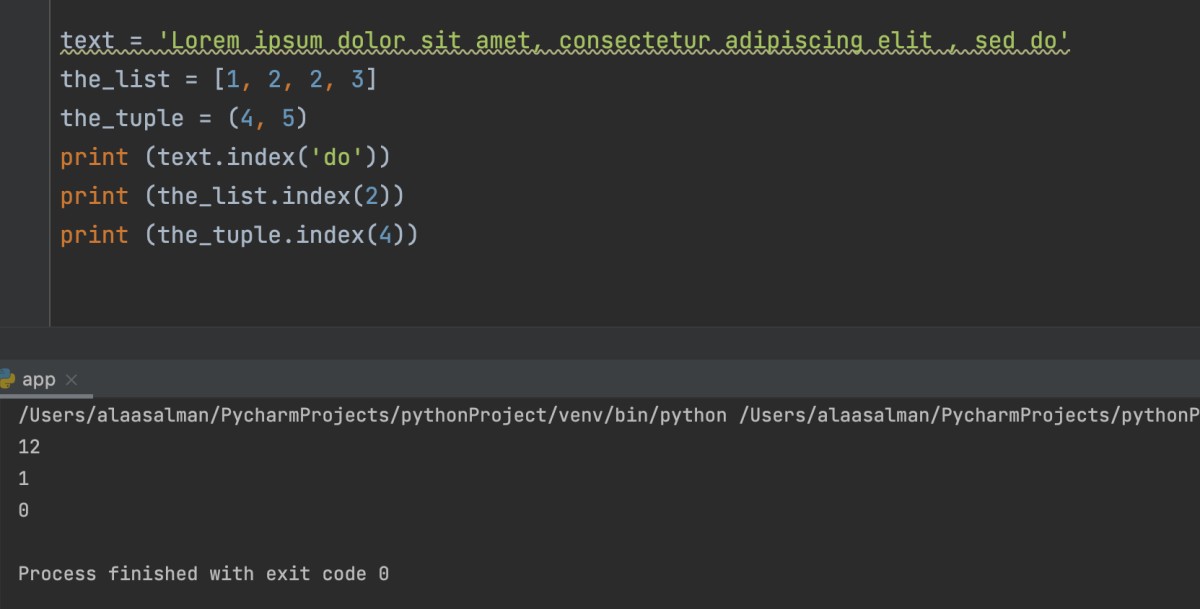
text = 'Lorem ipsum dolor sit amet, consectetur adipiscing elit , sed do'
the_list = [1, 2, 2, 3]
the_tuple = (4, 5)
print (text.index('do'))
print (the_list.index(2))
print (the_tuple.index(4))
Note: it takes the first element and ignores the rest.
What is Raw String?
when you prefix a string with the letter r or R such as r'...' and R'...', that string becomes a raw string. Unlike a regular string, a raw string treats the backslashes (\) as literal characters.
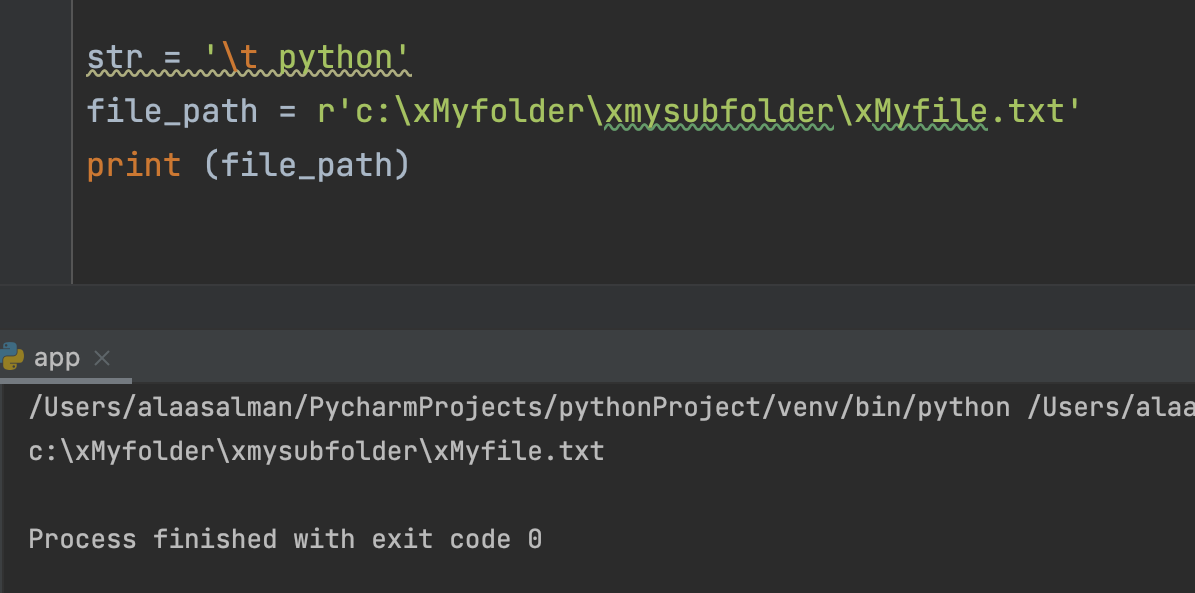
str = '\t python'
file_path = r'c:\xMyfolder\xmysubfolder\xMyfile.txt'
print (file_path)
List Comprehension
It offers a shorter syntax when you want to create a new list based on the values of an existing list.
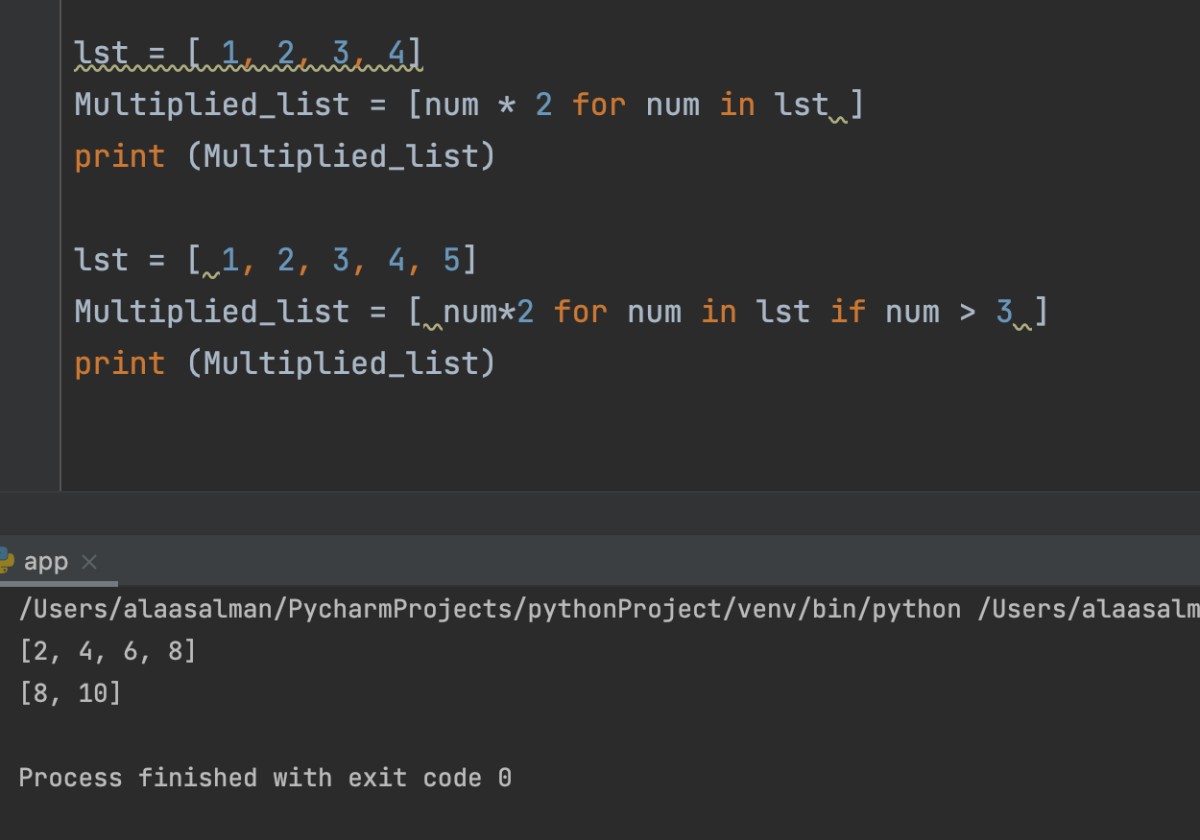
lst = [ 1, 2, 3, 4]
Multiplied_list = [num * 2 for num in lst ]
print (Multiplied_list)
lst = [ 1, 2, 3, 4, 5]
Multiplied_list = [ num*2 for num in lst if num > 3 ]
print (Multiplied_list)
What Are the Argument Types in Python
Python supports 3 types of arguments:
- Positional arguments/ required argument
- Default arguments / optional
- Keyword Arguments
What is positional/ required argument?
It is an argument that its position matters in a function call.
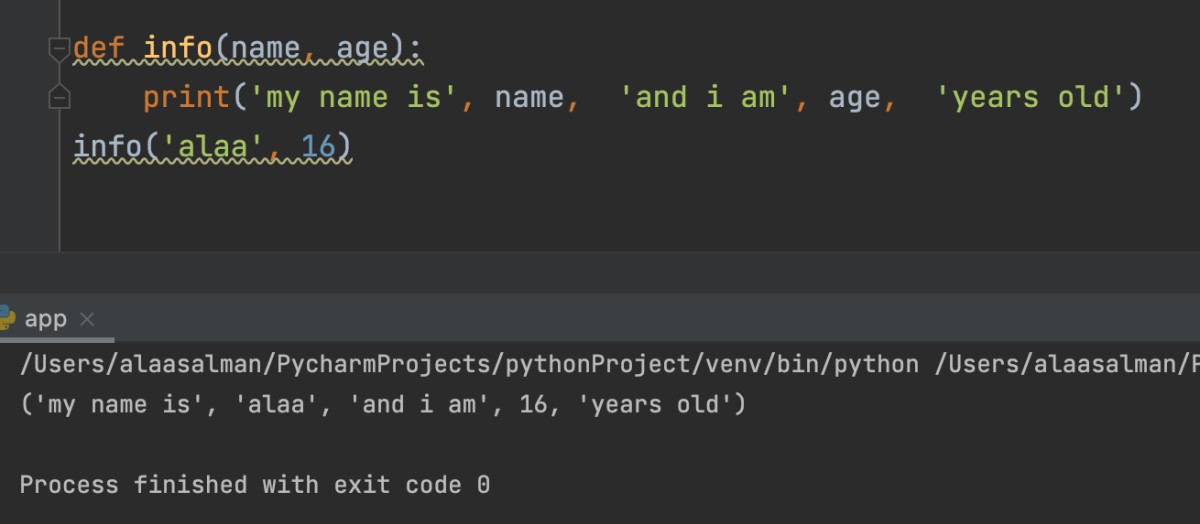
def info(name, age):
print('my name is', name, 'and i am', age, 'years old')
info('alaa', 16)
What is Default/ optional arguments?
Python allows function arguments to have default values. If the function is called without the argument, the argument gets its default value.
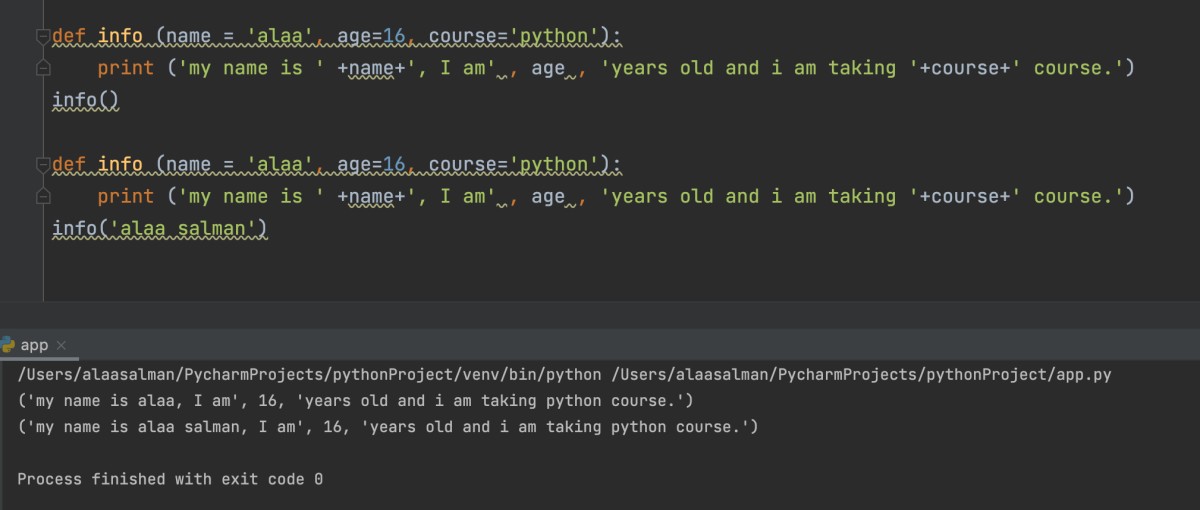
def info (name = 'alaa', age=16, course='python'):
print ('my name is ' +name+', I am' , age , 'years old and i am taking '+course+' course.')
info()
def info (name = 'alaa', age=16, course='python'):
print ('my name is ' +name+', I am' , age , 'years old and i am taking '+course+' course.')
info('alaa salman')
What is Keyword Arguments?
The order of the arguments does not matter.
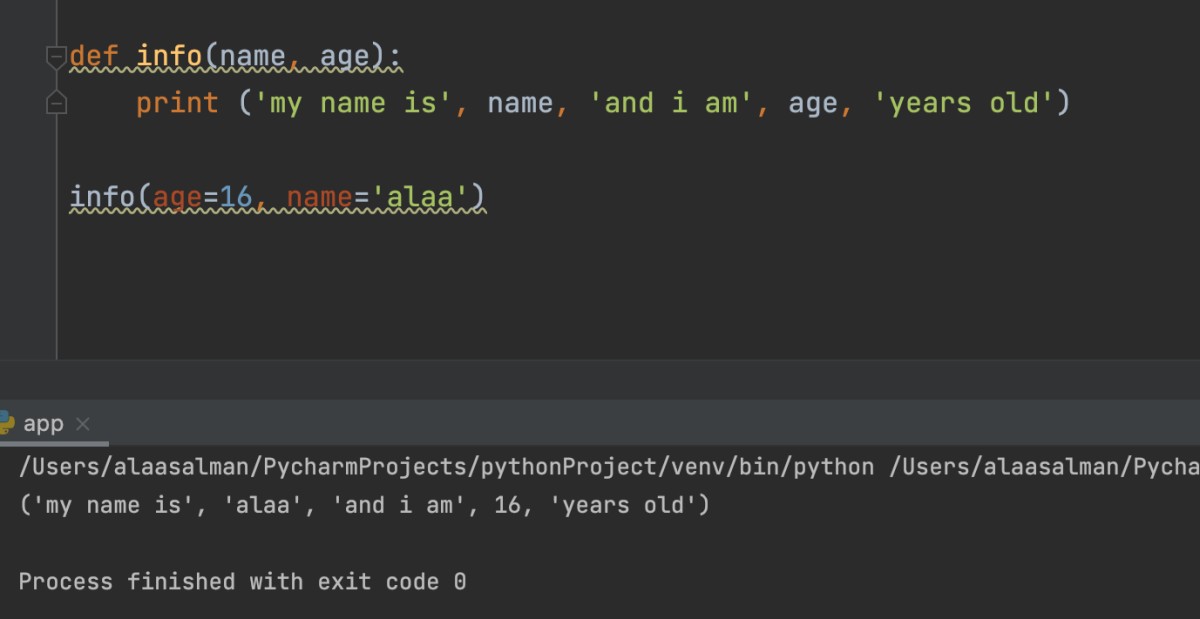
def info(name, age):
print ('my name is' , name, 'and i am' , age, 'years old' )
info (age = 16, name = 'alaa' )
Arguments Packing & Unpacking
What is an Arguments Packing?
*args and **kwargs , it’s known as packing, which packs all the arguments in a tuple. If we don’t know the number of arguments to be passed during the function call, we can use packing.
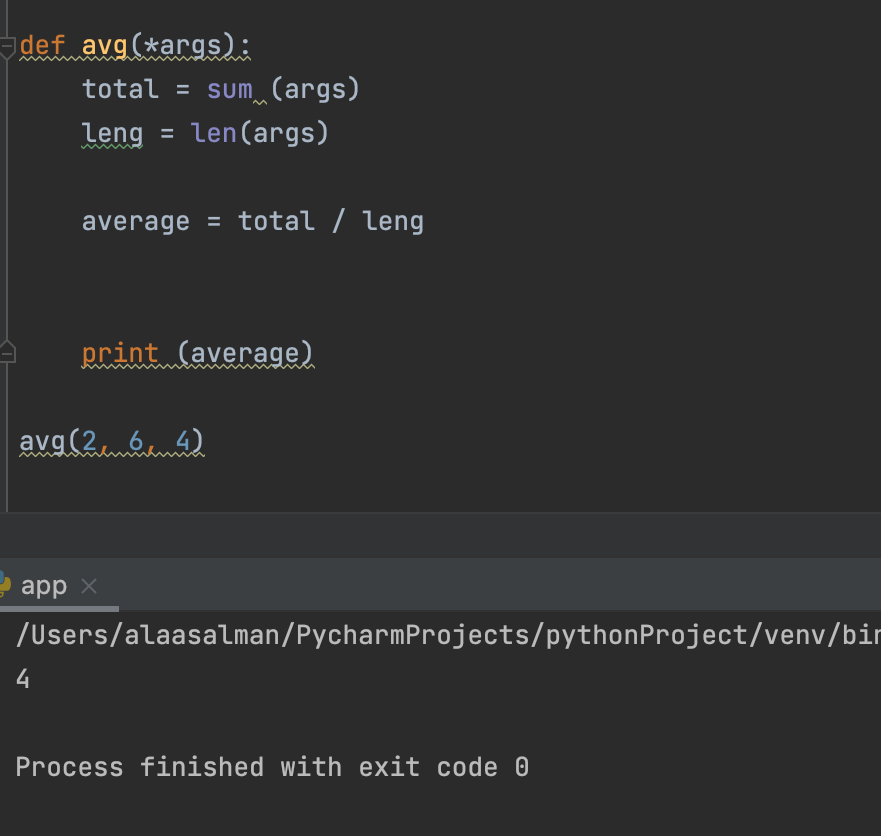
def avg(*args):
total = sum (args)
leng = len(args)
average = total / leng
print (average)
avg(2, 6, 4)
What is an Arguments UnPacking?
- During function call, we can unpack python list/tuple and pass it as separate arguments.
- It is used for unpacking positional arguments and keyword arguments.
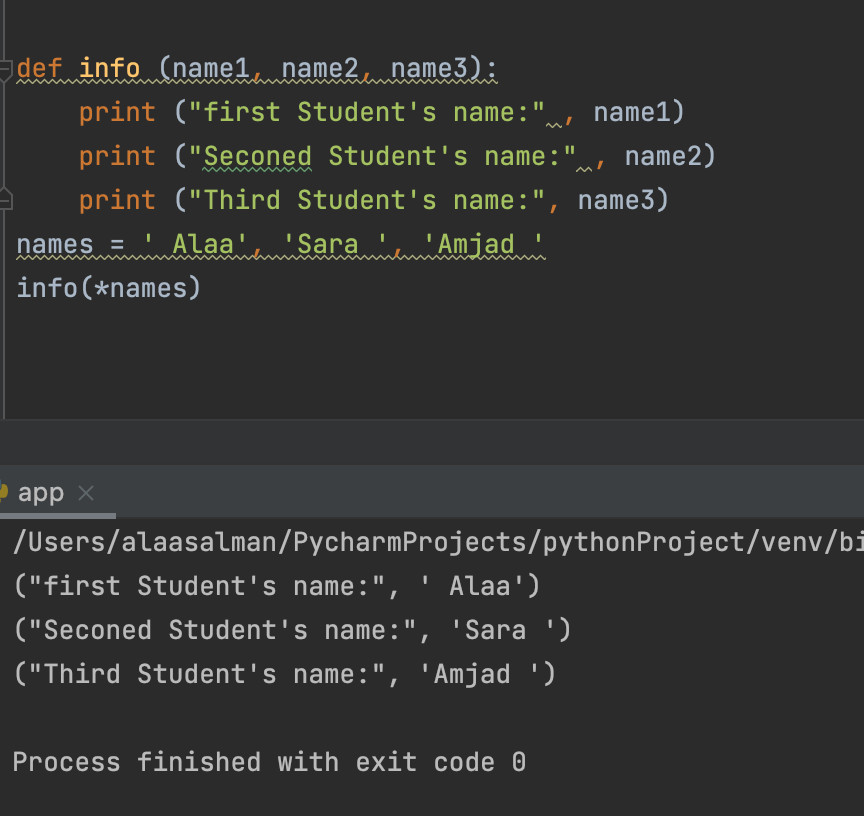
def info (name1, name2, name3):
print ("first Student's name:" , name1)
print ("Seconed Student's name:" , name2)
print ("Third Student's name:", name3)
names = ' Alaa', 'Sara ', 'Amjad '
info(*names)
What is Argument Dictionary Packing?
You can use **kwargs To apply Argument Dictionary Packing.
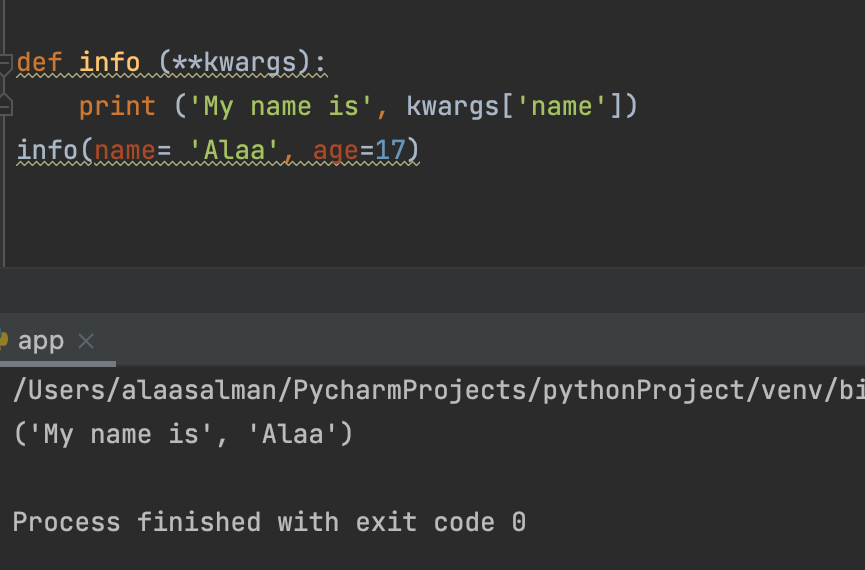
def info (**kwargs):
print ('My name is', kwargs['name'])
info(name= 'Alaa', age=17)
What is Argument Dictionary Unpacking?
You can use ** To apply Argument Dictionary UnPacking.
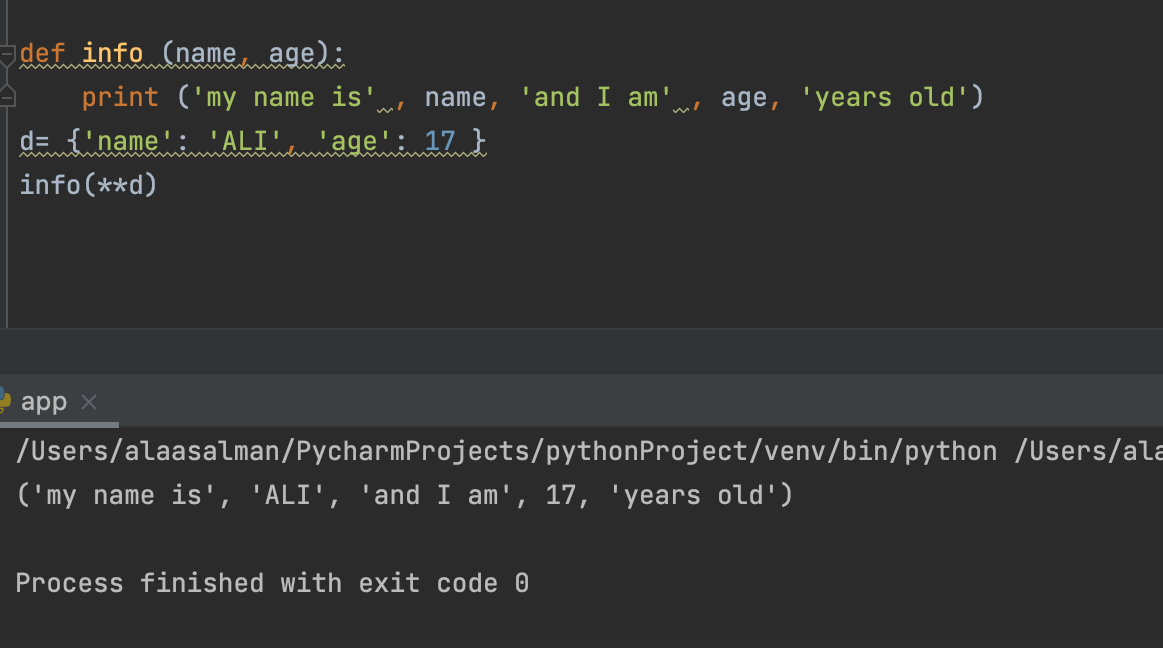
def info (name, age):
print ('my name is' , name, 'and I am' , age, 'years old')
d= {'name': 'ALI', 'age': 17 }
info(**d)
Creating the constructor in python
The method the __init__() simulates the constructor of the class. This method is called when the class is instantiated. It accepts the self-keyword as a first argument which allows accessing the attributes or method of the class.
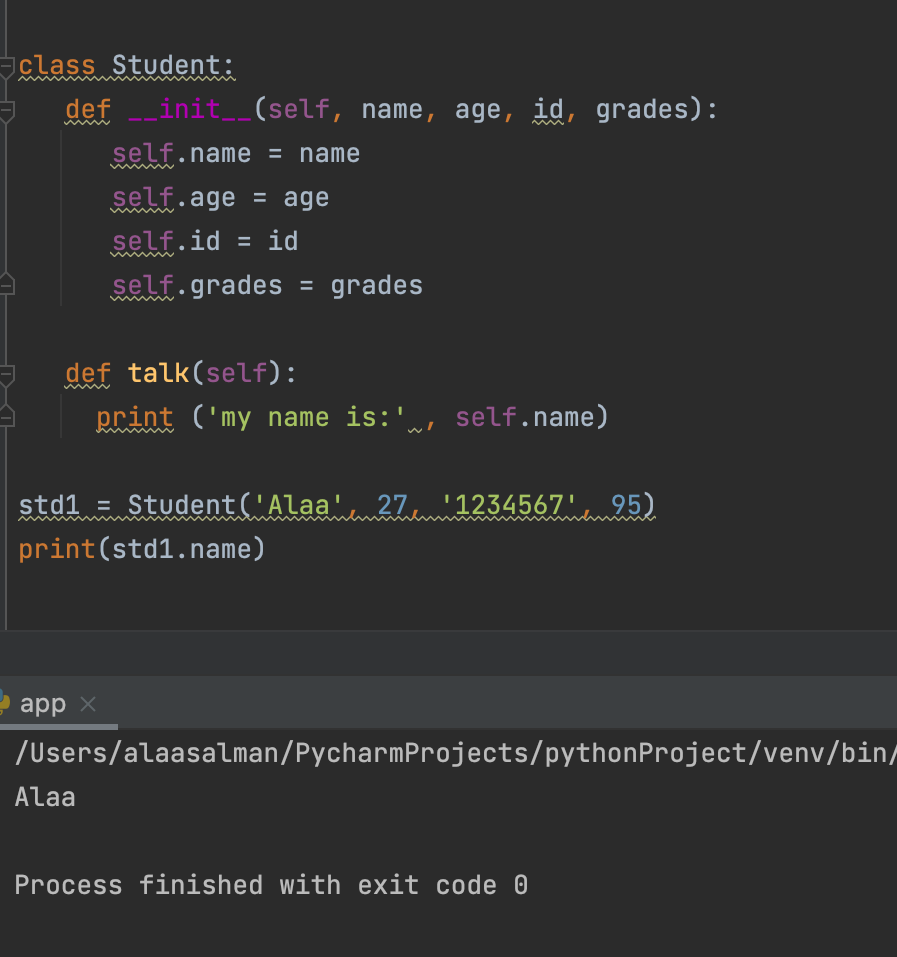
class Student:
def __init__(self, name, age, id, grades):
self.name = name
self.age = age
self.id = id
self.grades = grades
def talk(self):
print ('my name is:' , self.name)
std1 = Student('Alaa', 27, '1234567', 95)
print(std1.name)
What’s Encapsulation?
Encapsulation is a way to ensure security by Getters And Setters Method. Basically, it refers to the bundling of data, along with the methods that operate on that data, into a single unit.
What Are Getters And Setters
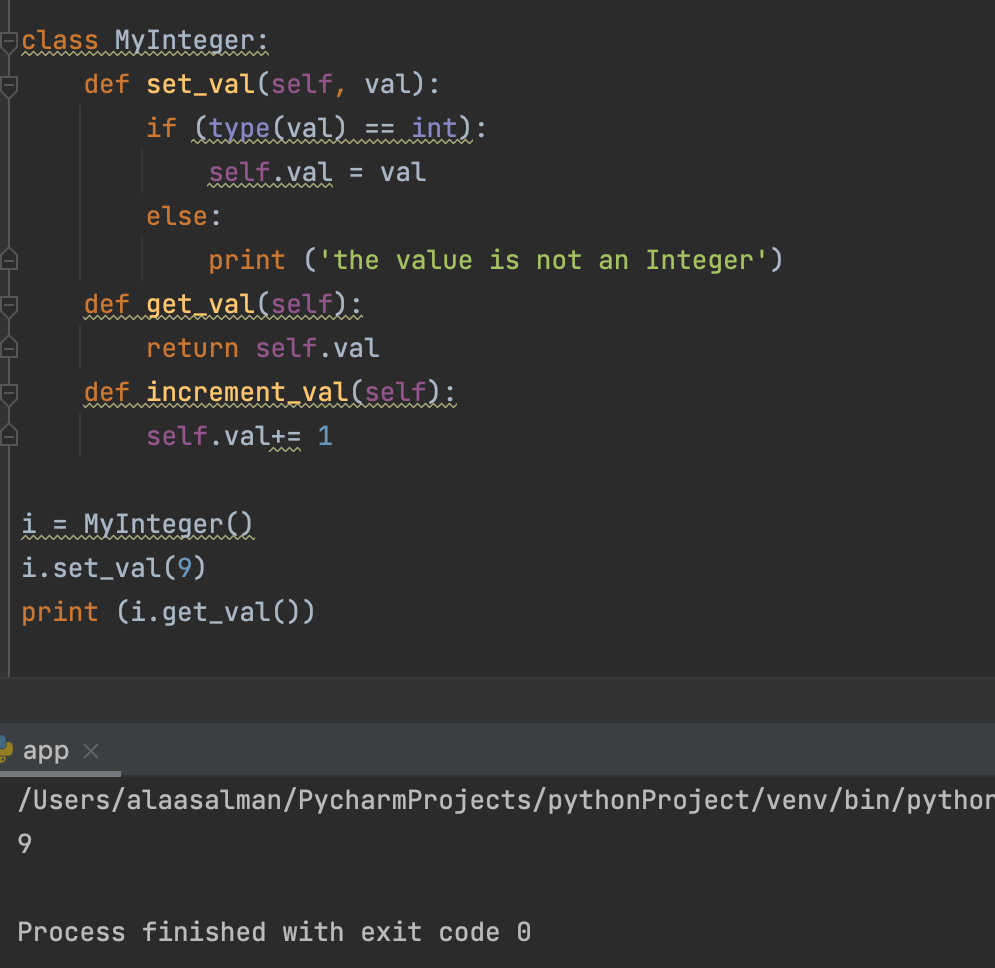
class MyInteger:
def set_val(self, val):
if (type(val) == int):
self.val = val
else:
print ('the value is not an Integer')
def get_val(self):
return self.val
def increment_val(self):
self.val+= 1
i = MyInteger()
i.set_val(9)
print (i.get_val())
Access modifiers:
A Class in Python has three types of access modifiers:
- Public Access Modifier
- Protected Access Modifier
- Private Access Modifier
What is public Access Modifier?
The members of a class that are declared public are easily accessible from any part of the program. All data members and member functions of a class are public by default , x=10.
What is protected Access Modifier?
The members of a class that are declared protected are only accessible to a class derived from it. Data members of a class are declared protected by adding a single underscore ‘_’ symbol before the data member of that class , _x=10.
What is private Access Modifier?
The members of a class that are declared private are accessible within the class only, private access modifier is the most secure access modifier. Data members of a class are declared private by adding a double underscore ‘__’ symbol before the data member of that class, __x= 10 .
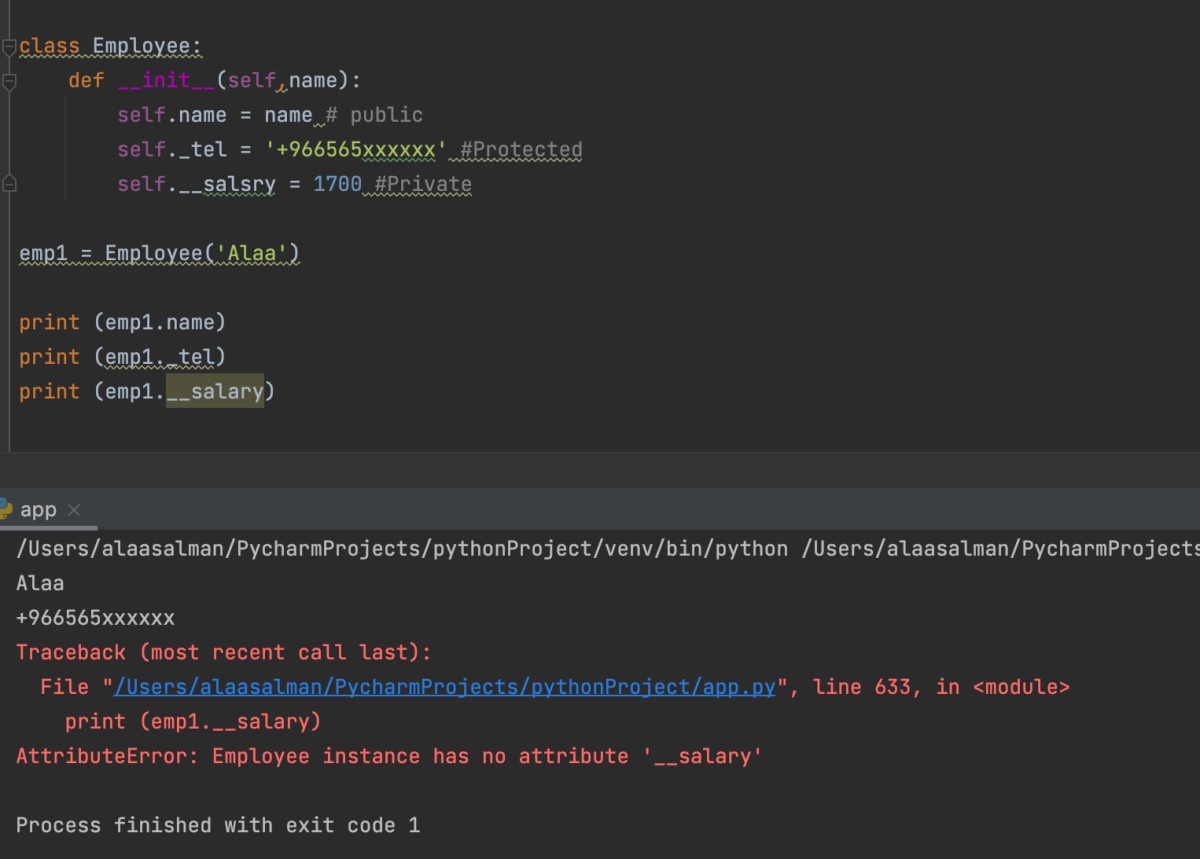
class Employee:
def __init__(self,name):
self.name = name # public
self._tel = '+966565xxxxxx' #Protected
self.__salsry = 1700 #Private
emp1 = Employee('Alaa')
print (emp1.name)
print (emp1._tel)
print (emp1.__salary)
Note: By get method, we can access to the private modifier
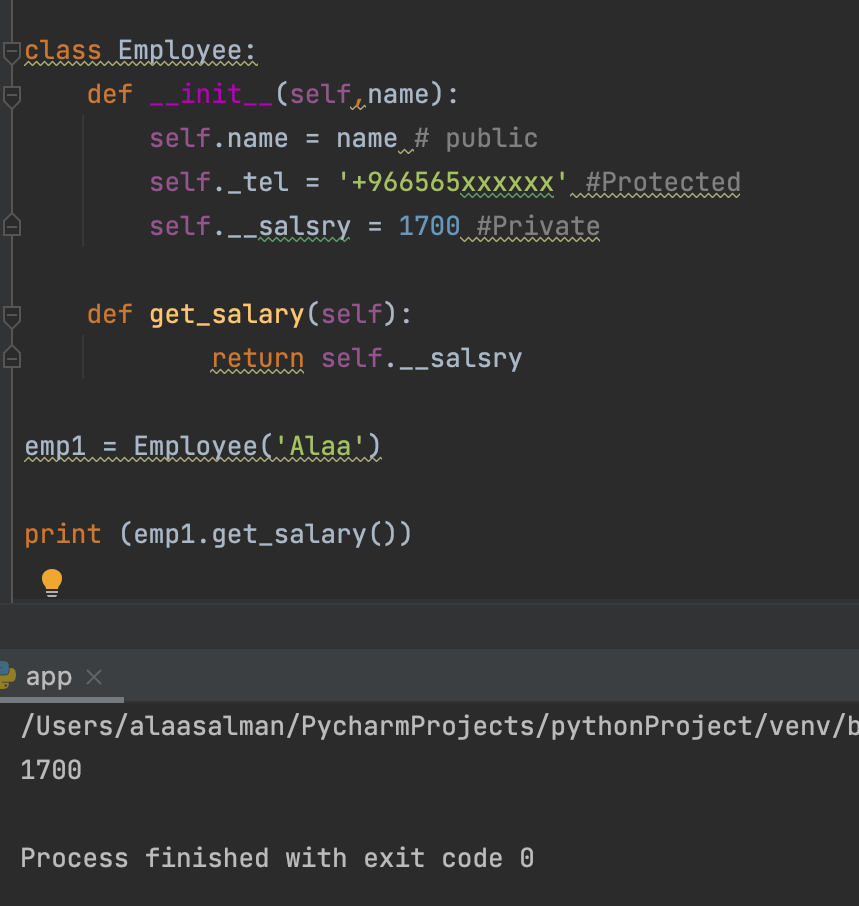
class Employee:
def __init__(self,name):
self.name = name # public
self._tel = '+966565xxxxxx' #Protected
self.__salsry = 1700 #Private
def get_salary(self):
return self.__salsry
emp1 = Employee('Alaa')
print (emp1.get_salary())
- How to assign new attributes?
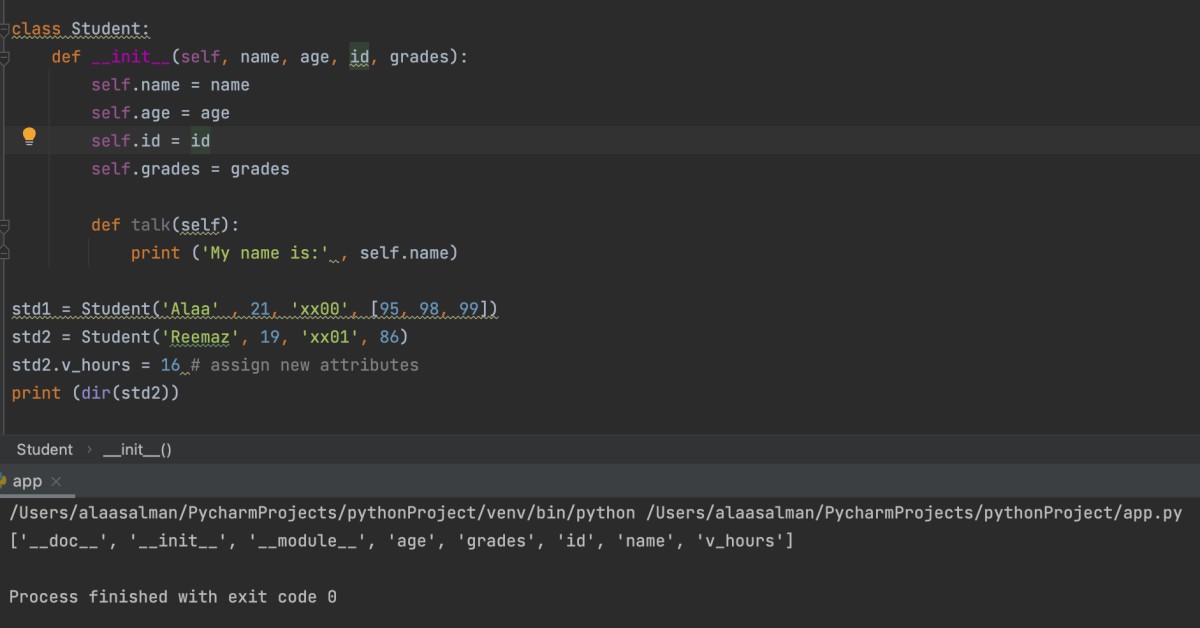
class Student:
def __init__(self, name, age, id, grades):
self.name = name
self.age = age
self.id = id
self.grades = grades
def talk(self):
print ('My name is:' , self.name)
std1 = Student('Alaa' , 21, 'xx00', [95, 98, 99])
std2 = Student('Reemaz', 19, 'xx01', 86)
std2.v_hours = 16 # assign new attributes
print (dir(std2))
- How to delate an attributes?
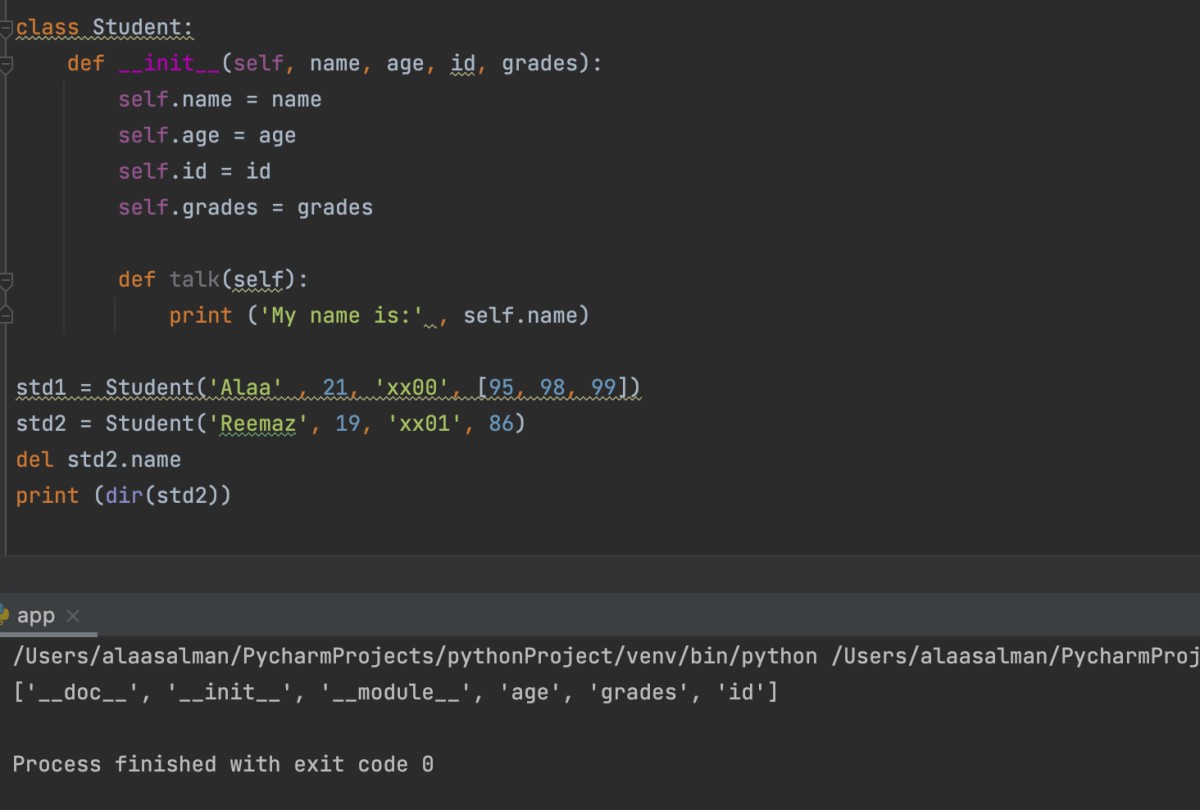
class Student:
def __init__(self, name, age, id, grades):
self.name = name
self.age = age
self.id = id
self.grades = grades
def talk(self):
print ('My name is:' , self.name)
std1 = Student('Alaa' , 21, 'xx00', [95, 98, 99])
std2 = Student('Reemaz', 19, 'xx01', 86)
del std2.name
print (dir(std2))
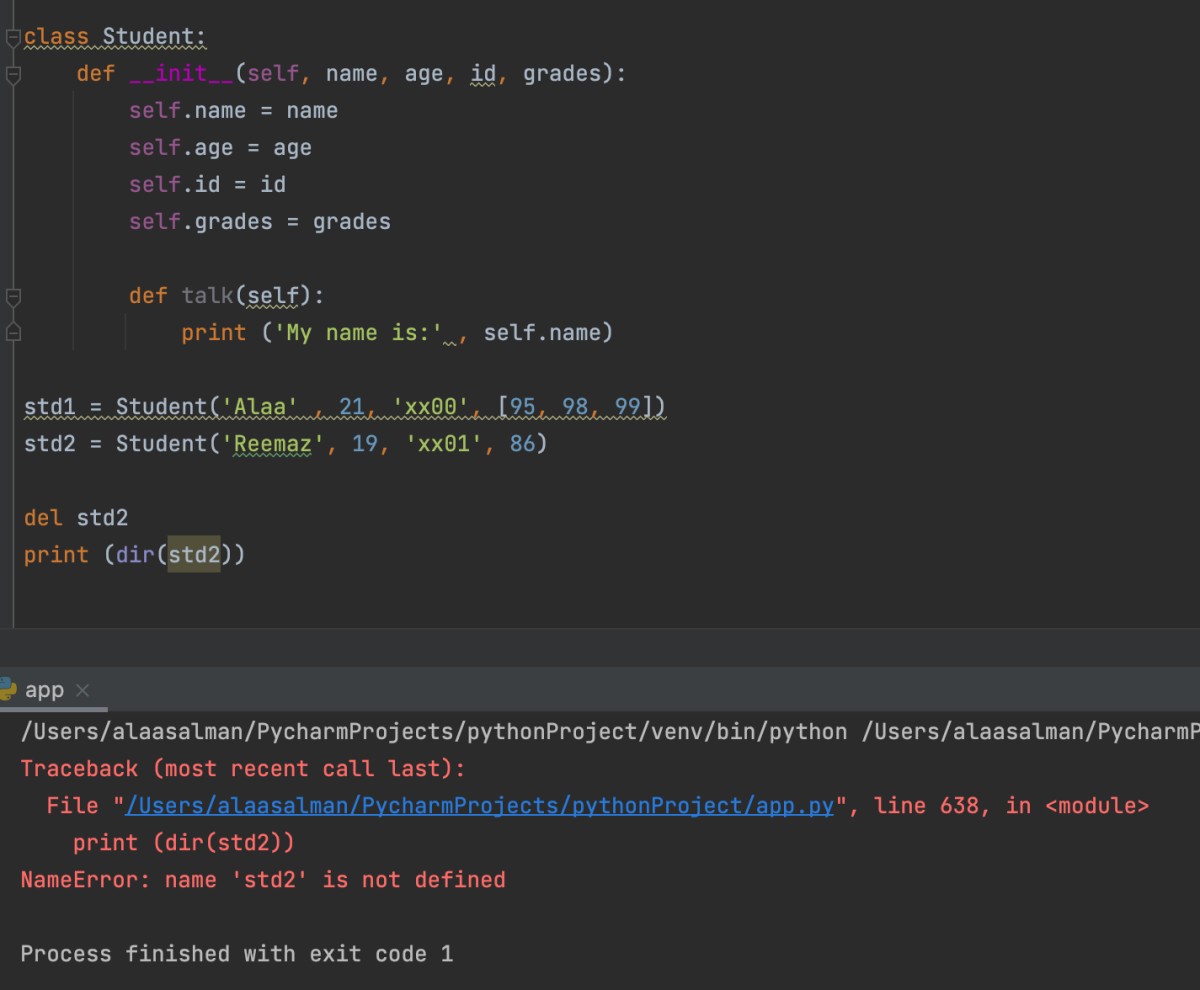
class Student:
def __init__(self, name, age, id, grades):
self.name = name
self.age = age
self.id = id
self.grades = grades
def talk(self):
print ('My name is:' , self.name)
std1 = Student('Alaa' , 21, 'xx00', [95, 98, 99])
std2 = Student('Reemaz', 19, 'xx01', 86)
del std2
print (dir(std2))
Conclusion
In this article, It has been discussed the Indexing vs Slicing, Raw String, List comprehension, 3 types of Arguments,Arguments Packing & Unpacking, How to create Constructor, What Are Getters And Setters and Access modifiers.