In this post, we're gonna learn about Switch statement in
Before we getting started, let's first know more about Switch statement in Programming Languages
What is a Switch statement?
- Switch statement is a multiway branch statement.
- Switch statement is a selection statement that chooses one section to execute from its cases that match the expression.
- Switch statement is used as an alternative of if-else statement.
- You can have any number of case statements within a switch.
Switch statement in C#
- The switch expression is evaluated once.
- Only one switch section in a switch statement executes.
- The data type of the variable in the switch and value of a case must be of the same type.
- The break in switch statement is used to terminate the current sequence.
- C# doesn't allow execution to continue from one switch section to the next, it must contain a jump statement.
- When the expression matches the case value the block of code will be executed.
Switch Syntax in C#
switch(expression)
{
case x:
// code block
break;
case y:
// code block
break;
.
.
case N:
// code block
break;
default:
// code block
break;
}
Example of the switch statement in C#
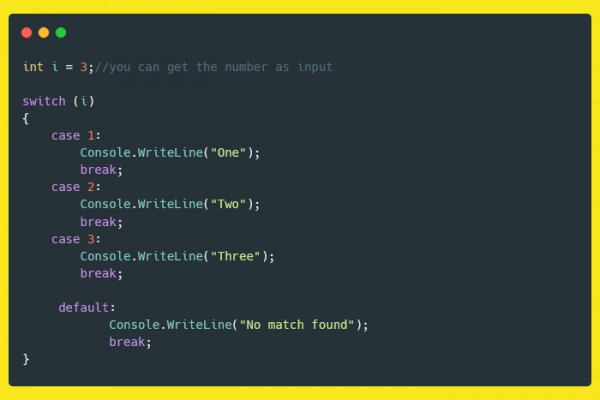
Switch statement in Python
- Python does not have a switch or case statement.
- To implement switch statement in Python is to use the built-in dictionary mappings.
- It can be implemented using an if-elif-else statement.
Example using a dictionary to implement switch statement
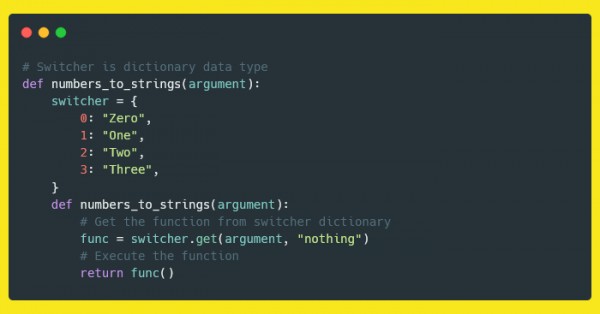
Switch statement in Java
- switch statement is a selection mechanism used to allow the value of an expression to change the flow of the program execution.
- Duplicate cases value is not allowed.
- The data type of the variable in the switch and value of a case must be of the same type.
- The break in switch statement is used to terminate the current sequence.
- Unlike the C#,the break statement is optional if not found the execution will continue to the next case;
Example of switch statement in java
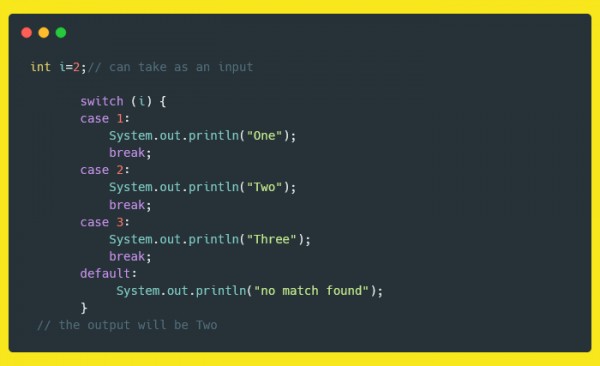
Here is an example that shows that the break statement is optional to use
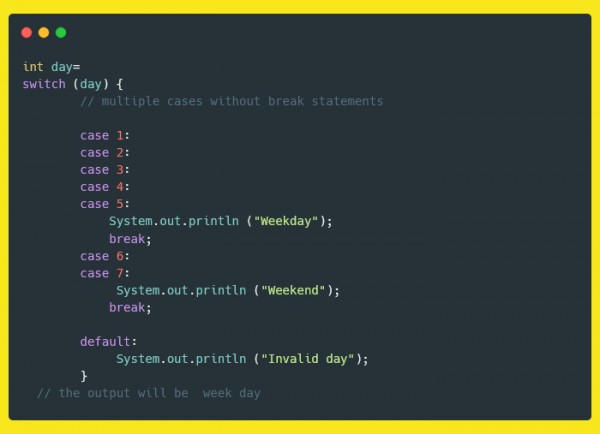
See Also