In this post, we're gonna learn about strings in C#.
What's "string"?
- string used to represent text.
string is a data type using in programming languages like integers and other data types.- string is a sequence of characters that may contain spaces and numbers within it.
strings in C#
C# represents "string" as a collection of characters surrounded by double quotes " ".
we can define a variable from type string as below:
string str = "Hello";
we can also use "string" as an object that contains methods and properties.
str.Length
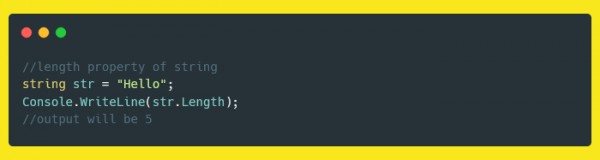
Length is used to get the number of characters that are in the string.
string methods
In this section, we will list here some of the string methods with examples in c#.
ToUpper()
ToUpper() converts String into Upper case.
Ex:
string firstName ="John";
string lastName ="Steven";
//ToUpper() Converts String into Upper case .
Console.WriteLine(firstName.ToUpper());
//output will be JOHN
ToLower()
ToLower() converts String into lower case.
Ex:
Console.WriteLine(firstName.ToLower());
//output will be john
Equals()
Equals() compares two string and returns Boolean value.
Ex:
Console.WriteLine(firstName.Equals(lastName));
//output will be false
EndsWith()
EndsWith() check if a string end with a specified character.
Ex:
Console.WriteLine(firstName.EndsWith("n"));
//output will be true
IndexOf()
IndexOf() return the first index of a specified character.
Ex:
Console.WriteLine(firstName.LastIndexOf("h"));
//the output will be 2
Insert()
Insert() insert a character or string in a specified position.
Ex:
Console.WriteLine(firstName.Insert(0, "Hello"));
//the output will be Hello john
LastIndexOf()
LastIndexOf() return last occurrence of specified character.
Ex:
Console.WriteLine(lastName.LastIndexOf("e"));
//output will be 4
Remove()
Remove() delete all characters from a specified index of a string.
Ex:
Console.WriteLine(firstName.Remove(2));
//the output will be jo
Split()
Split() split a string based on a specific delimiter.
Ex:
In this example, we will use the split method to return an array of strings separated by "a comma followed by space" as a delimiter.
string colorList="red, yellow, black";
string[] colorName = colorlist.Split(", ");
foreach (string ColorName in colorList)
Console.WriteLine(ColorName);
//the output will be
/* red
yellow
black */
Substring()
Substring() is a string method that used to return a substring from the main string by specifying two parameters:
- Start Position.
- The character's length.
Ex:
Console.WriteLine(firstName.Substring(1,3));
// the output will be ohn
ToCharArray()
ToCharArray() converts string into char array.
Ex:
Console.WriteLine(firstName.ToCharArray());
//the output will be John
Conclusion
In this post, we have explained the String in C# programming language, we have also explored some of the important string methods.
- ToUpper()
- ToLower()
- Equals()
- EndsWith()
- IndexOf()
- Insert()
- Remove()
- Split()
- Substring()
- ToCharArray()
See Also