Consume a REST API in MVC Step By Step
To Consume a REST API with Different Action (GET and POST) in MVC Application, you should follow the below steps in details:
- First, Create an API project within the Same Solution.
- Create an API with a name
show-signature
and its Route is : /api/APPLICATION_NAME/show-signature
.
- Navigate to the Web.config file and access the appSettings section.
- Add in appSettings 2 key and it's value
- Key
RemoteBaseURL
and value is http://localhost:5000/
- Key
RemoteBaseApiURL
and value is api/APPLICATION_NAME/
- Create a class to act as a Middleware to
Intercept
API Calls in your MVC Application called CustomRemoteActions
.
- Invoke the "
ShowSignatue
" , "Print
" and "UploadSignature
" Method in an MVC Controller,
- Read the Response Using
ReadAsStringAsync
,
- Finally Deserialize it into a Custom Class You've Created.
Examples
1) Considering that the API has been created either by you or integrated with any sector .
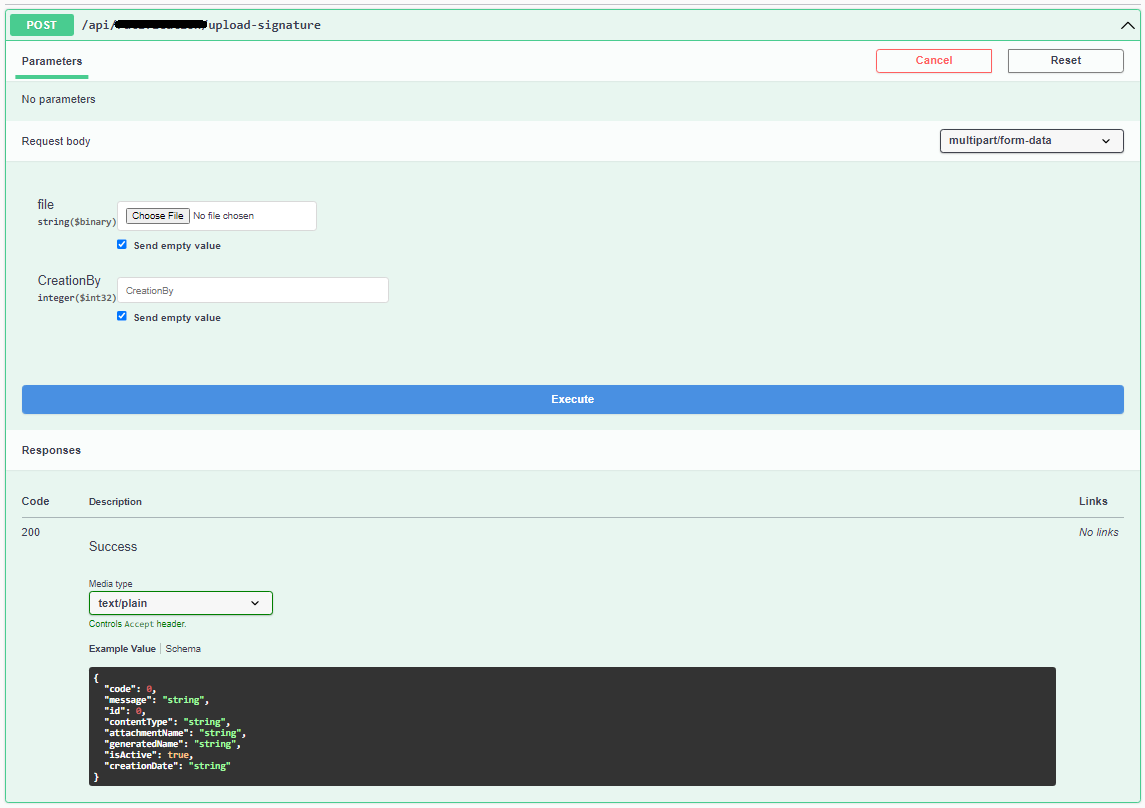
2) Add in appSettings 2 key and it's value in Web.Config
<appSettings>
<add key="RemoteBaseURL" value="http://localhost:5000/" />
<add key="RemoteBaseApiURL" value="api/APPLICATION_NAME/" />
</appSettings>
3) Extract values for RemoteBaseURL and RemoteBaseApiURL from the Web.Config configuration .
static string RemoteBaseURL = ConfigurationManager.AppSettings["RemoteBaseURL"];
static string RemoteBaseApiURL = ConfigurationManager.AppSettings["RemoteBaseApiURL"];
4) Perform a GET Request to an Action into CustomRemoteActions
class in mvc application that consume API from previous swagger
public static HttpResponseMessage ShowSignatue(string endpoint)
{
var getToken = CreateToken().Result;
string BaseUrl = string.Format("{0}{1}{2}", RemoteBaseURL, RemoteBaseApiURL, endpoint);
HttpResponseMessage response;
using (HttpClient client = new HttpClient())
{
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", getToken.access_token);
response = client.GetAsync(BaseUrl).Result;
if (response.IsSuccessStatusCode)
{
return response;
}
else
{
throw new Exception("يوجد مشكلة في الاتصال الخارجي بالنظام");
}
}
}
5) Deserialize object Method
var signatue = CustomRemoteActions.ShowSignatue("show-signature");
var cu = signatue.Content.ReadAsStringAsync()?.Result;
SignatureModel vm = JsonConvert.DeserializeObject<SignatureModel>(cu);
6) Perform a POST Request to an Action into CustomRemoteActions
class in mvc application that consume API from previous swagger
public static HttpResponseMessage Print(string endpoint, string ApplicationId,string Email, string MobileNo, int EmployeeId)
{
var getToken = CreateToken().Result;
string BaseUrl = string.Format("{0}{1}{2}", RemoteBaseURL, RemoteBaseApiURL, endpoint);
HttpResponseMessage response;
using (HttpClient client = new HttpClient())
{
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", getToken.access_token);
object data = new
{
ApplicationId = ApplicationId,
Email = Email,
MobileNo = MobileNo,
EmployeeId = EmployeeId
};
var json = JsonConvert.SerializeObject(data);
var stringContent = new StringContent(json, UnicodeEncoding.UTF8, "application/json");
try
{
response = client.PostAsync(BaseUrl, stringContent).Result;
}
catch (Exception ex)
{
return new HttpResponseMessage();
}
if (response.IsSuccessStatusCode)
{
return response;
}
else
{
return new HttpResponseMessage();
}
}
}
7) Perform a POST Request that contain files to an Action into CustomRemoteActions
class in mvc application that consume API from previous swagger
public static HttpResponseMessage UploadSignature(SignatureModel model)
{
try
{
var getToken = CreateToken().Result;
string BaseUrl = string.Format("{0}{1}{2}", RemoteBaseURL, RemoteBaseApiURL, "upload-signature");
HttpResponseMessage response;
using (HttpClient client = new HttpClient())
{
client.DefaultRequestHeaders.Clear();
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", getToken.access_token);
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
client.DefaultRequestHeaders.TryAddWithoutValidation("Content-Type", "multipart/form-data");
using (var formControl = new MultipartFormDataContent("NKdKd9Yk"))
{
var values = new[]
{
new KeyValuePair<string,string>("CreationBy",model.CreationBy.ToString())
};
foreach (var item in values)
{
formControl.Add(new StringContent(item.Value), item.Key);
}
formControl.Headers.ContentType.MediaType = "multipart/form-data";
StreamContent streamContent = new StreamContent(model.file.InputStream);
formControl.Add(streamContent, "file", model.file.FileName);
//log formcon
response = client.PostAsync(BaseUrl, formControl).Result;
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("multipart/form-data"));
if (response.IsSuccessStatusCode)
{
return response;
}
else
{
return new HttpResponseMessage();
}
}
}
}
catch (Exception ex)
{
throw new Exception("يوجد مشكلة في الاتصال الخارجي بالنظام");
}
}
See Also