In this post, we will explain one of the known Liner data structures which are Array, also When to use, and how to use it in C#.
What is Array?
The array is a linear data structure that is a collection of items stored in memory. The idea is to store multiple items of the same type together.
Syntax:
DataType[] arrayName;
How does Array work in Data Structure?
Array consists of two main sections
- Index, and
- Element.
What's Element in Array in Data Structure?
- Element has a specific value and data type, like integer 123 or string “hello”.
- Each element has its index, used to access the element. Index starts with 0.
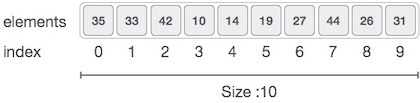
As you see in the above figure, this array is a collection of integer types and the index starts with 0.
Note: index starts with zero because the address in the memory starts with zero. For example, the location in our array is 5000 in the memory, the first element that index starts zero will be stored in 5000 because 5000+0 = 5000 and the second which index is 1 stored in 5000+1 = 5001, etc...
When to use Array in Data Structure?
In Data Structure, you may need to use the Array in the following cases:
- You need random access to elements.
- You know the number of elements in the array.
- Save memory. Arrays take less memory than linked lists.
Example: Implementing Code of Array in C#.
In this code, we will define an array and store five numbers in the array and then print each element by for-loop.
int[] numbers = { 13, 28, 35, 32, 7};
for (int i = 0; i < numbers.Length; i++)
{
Console.WriteLine(numbers[i]);
}
Output:
13
28
35
32
7
What is a two-dimensional Array?
C# supports multiple-dimensional. The multidimensional array can be declared by adding commas in the square brackets. For example, [ , ] declares two-dimensional, [ , , ] declares three-dimensional, etc...
In this section, we will focus on a two-dimensional array which can be thought of as a table, which has x number of rows and y number of columns. For example, we have in the figure three rows and two columns.
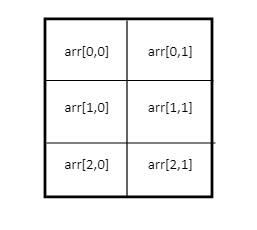
Syntax:
int[,] arr = new int[3, 2];
- three is the number of rows,
- two is the number of columns.
Example: Implementing Code of two-dimensional Array in C#.
In this code, we will define a two-dimensional array and store six numbers in the array.
int[,] arr = new int[3, 2] { {1, 2}, {3, 4}, {5, 6} };
arr[0, 0]; // returns 1
arr[0, 1]; // returns 2
arr[1, 0]; // returns 3
arr[1, 1]; // returns 4
arr[2, 0]; // returns 5
arr[2, 1]; // returns 6
arr[3, 0]; // returns 7
arr[3, 1]; // returns 8
Source code:
References: