In this post, we will discuss about Stack, which is one of the known types in Linear Data Structure using C#.
What is Stack in data structure?
A Stack is a linear data structure, that follows a particular order named LIFO stands for Last-In-First-Out which means the last item in the list is the first item to out.
Stack has two primary operations to handle the list:
- Insertion operation called PUSH, and
- Removal or Deletion operation called POP.
The below figure explains these two operations.
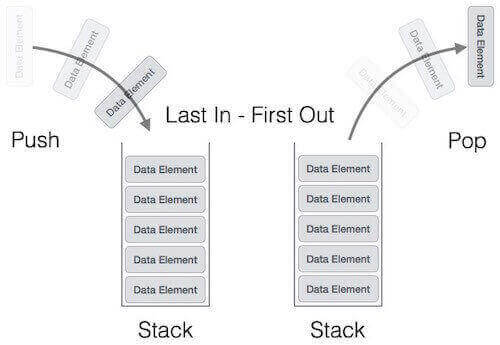
Key points of the stack:
- It is called Stack because it behaves like a real-world Stack, like a Stack of papers.
- A Stack is an abstract data type with a pre-defined capacity, which means that it can store the elements of a limited size.
- It is a data structure that follows an order for insert and remove and this order is called LIFO.
How does stack work in data structure?
A Stack is a very simple data structure, as we see before in #primary operations the working of Stack as a data structure can be understood through these operations.
Let’s explain each of them one by one.
1. Insertion Operation (push)
The insert to an element in a data structure called “Push” operation.
When we insert it into the stack, it occupies the bottom-most position. The next element inserted goes to the top of the previous element and likewise, the insertion of all elements happens. As each time, the insertion operation resembles pushing an element into the stack.
2. Removal or Deletion Operation (pop)
- The operation to remove an element from the Stack is called the “Pop” operation.
- The pop operation removes the most recently added element first.
And the below figure explains more about these two operations.
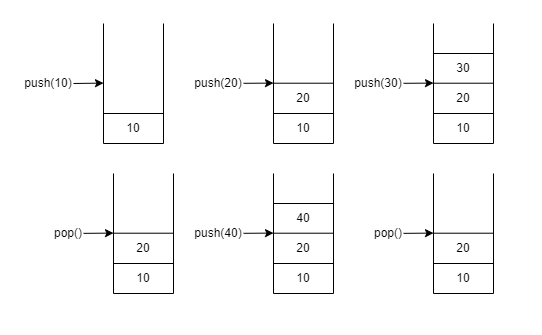
3. Top Operation
Here we have the third operation it is not important like previous operations, but still it is useful, and like it is named it returns the top of the Stack which is the last element inserted in the Stack. For example, if insert in the Stack numbers: 1, 2, 3, 4, 5 and the last element inserted is five, if we use the top method it will return the last element which is five. And in C# top method called peek().
This animation explains how dose stack works:
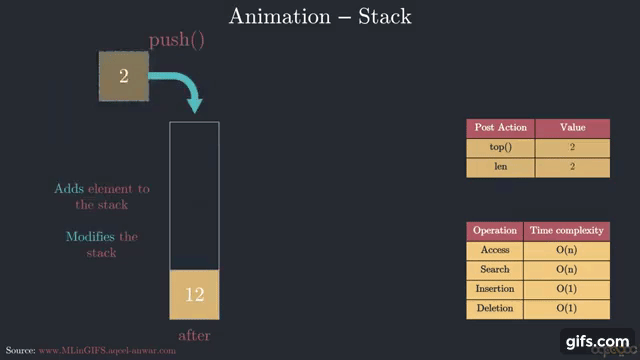
When to use the stack in data structure?
Here are some common applications in stack Data Structure is used:
- Reversing: when you want to get things out in the reverse order.
- Undo/redo: when you want to implement backward and forward functionality. For example, the text editor has undo and redo.
- In browser: the button in the browser saves all the URLs you have visited. When pressing the back button, it is POP from the stack the last URL and the top URL is accessed.
Example: Implementing Code of the stack in C#
In this example, you will learn how to create a Stack, how to insert an element and remove it, and search the last element inserted in the Stack.
var stack = new Stack();
stack.Push(10); // insert elements in the stack
stack.Push(20);
stack.Push(30);
stack.Push(40);
Console.WriteLine("Stack elements...");
Console.WriteLine("the count of elements: " + stack.Count);
Console.WriteLine("the top element in the stack is: " + stack.Peek());
stack.Pop(); // remove the last element inserted in the stack
Console.WriteLine("the count of elements (updated) : " + stack.Count);
Console.WriteLine("the top element in the stack is (updated) : " + stack.Peek());
foreach (var item in stack)
Console.WriteLine(item);
stack.Clear(); // this remove all elements in the stack
if(stack.Count == 0)
Console.WriteLine("stack is empty plese insert some elements.");
Output
Stack elements...
the count of elements: 4
the top element in the stack is: 40
the count of elements (updated) : 3
the top element in the stack is (updated) : 30
30
20
10
Source code
you can get the source code from GitHub by clicking here!
References: