In this article, we will take a brief about Design Patterns and their types and we will dig deeper into one of the popular types the Singleton Design Pattern, then why use Singleton, the last one the implementation of the Singleton Design Patterns.
What are Design Patterns?
Design Patterns are solutions to software design problems you find again and again in real-world application development. Patterns are about reusable designs and interactions of objects.
It’s isn't a finished design that can be transformed directly into code. It is a description or template for how to solve a problem that can be used in many different situations.
The UML of Singleton Design Pattern
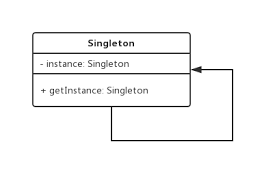
GoF Design Pattern Types
Gang of Four (GoF) Design Patterns are divided into three categories:
- Creational: The patterns that provide a way to create objects while hiding the creational logic, this provides us flexibility for creating objects based on different use cases.
- Structural: The patterns in this category deal with the class structure such as Inheritance and Composition.
- Behavioral: The patterns that are concerned with communication between objects hence increasing flexibility between objects.
What are Singleton Design Patterns?
Singleton Design Pattern in C# is one of the most popular design patterns. And Singleton is one of the Creational types. In this pattern, a class has only one instance in the program that provides a global point of access to it.
In other words, a Singleton is a class that allows only a single instance of itself to be created.
Why use Singleton Design Patterns?
The Singleton Pattern solves two problems at the same time, violating the Single Responsibility Principle:
1) Ensure that a class has just a single instance.
The most common reason for this is to control access to some shared resource. And this instance is only reachable by the static method. For example database or file.
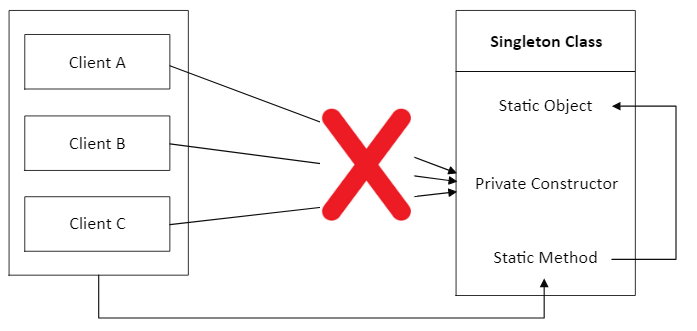
2) Provide a global access point to that instance.
Just like a global variable, the Singleton pattern lets you access some object from anywhere in the program. However, it also protects that instance from being overwritten by other codes.
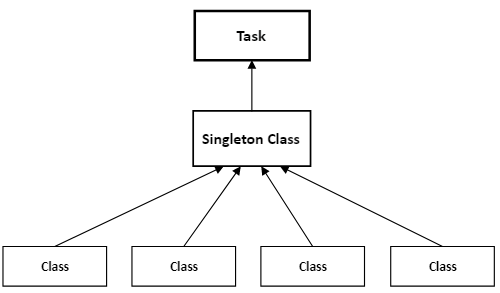
Implementation of the Singleton Design Patterns in C#
There are various ways to implement a Singleton Pattern in C#. And below is the common way to implement these patterns.
- Sealed class.
- Private and parameter-less single constructor.
- Static and private variable to hold a reference to the single created instance
- A public and static way of getting the reference to the created instance.
public sealed class Singleton
{
// The Singleton's constructor should always be private.
private Singleton() { }
// The Singleton's instance is stored in a static field.
private static Singleton _instance;
// This is the static method that controls the access to the singleton
// instance. On the first run, it creates a singleton object and places
// it into the static field.
public static Singleton GetInstance()
{
if (_instance == null)
_instance = new Singleton();
return _instance;
}
}
class Program
{
static void Main(string[] args)
{
var s1 = Singleton.GetInstance();
var s2 = Singleton.GetInstance();
if (s1 == s2)
Console.WriteLine("Singleton works, both variables contain the same instance.");
else
Console.WriteLine("Singleton failed, variables contain different instances.");
// hash code may be different from your machine but should be both equal to each other.
Console.WriteLine("\nHash code of S1: " + s1.GetHashCode());
Console.WriteLine("Hash code of S2: " + s2.GetHashCode());
}
}
Expected output
Singleton works, both variables contain the same instance.
Hash code of S1: 58225482
Hash code of S2: 58225482
References
- Singleton - refactoring.guru
- Singleton Design Pattern In C# - c-sharpcorner