In this article, we will understand What is class and the types of classes in C#, and we will also dig deeper into each type by knowing the key points and differences between Abstract Class Vs Partial Class Vs Static Class Vs Sealed Class
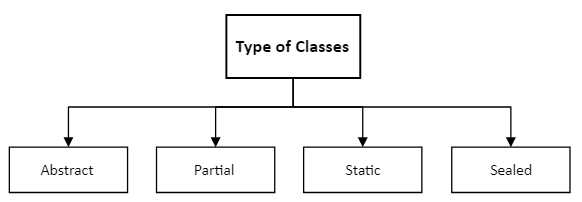
What is Class?
Everything in C# is associated with classes and objects, with its attribute and methods. Class and object are the essential components in object-oriented programming.
For example: in real life, a car is an object also there is attribute like color and weight. And the methods such as driving forward, driving backward, and breaking.
public class Car
{
// Attribute
string color = "blue";
int weight = 2000;
// Methods
public string DriveForward()
{
return "drive forWard";
}
public string DriveBackward()
{
return "drive backWard";
}
}
Some key points about classes
- Classes are reference types that hold the object created dynamically in a heap.
- The default access modifier of a class is Internal.
- The default access modifier of methods and variables is Private.
- Directly inside the namespaces declarations of private classes are not allowed.
Type of Classes?
In C#, there are four types of classes which are:
- Abstract Class
- Partial Class
- Static Class
- Sealed Class
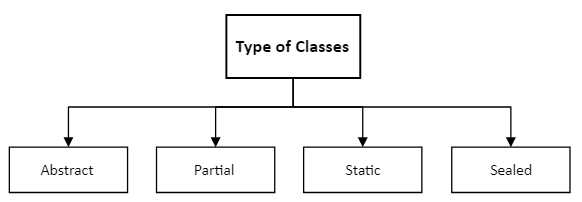
In the next section, we will understand each class type one by one by giving the definition and when to use it then the key points of each type.
What is Abstract Class?
Abstraction is the process of hiding certain details and showing only essential information to the user.
Abstract Class is a restricted class that cannot be used to create objects (to access it, it must be inherited from another class).
When to use Abstract Class?
- It’s also good if you want to declare non-public members.
- Abstract Classes are a good fit if you want to provide implementation details to your children but don't want to allow an instance of your class to be directly instanced.
Abstract Class Key points
- It is declared using the abstract keyword.
- Cannot create an object.
- If you want to use it then it must be inherited.
- An Abstract class contains both abstract and non-abstract methods.
- An Abstract class has only one subclass.
- Methods inside the abstract class cannot be private.
- Methods in abstract class don’t have a body.
- If there is at least one method abstract in a class then the class must be abstract.
Example
public abstract class drow
{
public abstract void draw();
}
class Circle : drow
{
public override void draw()
{
Console.WriteLine("draw Circle");
}
}
class Cube : Circle
{
public override void draw()
{
Console.WriteLine("draw Cube");
}
}
class Program
{
static void Main(string[] args)
{
var circle = new Circle();
var cube = new Cube();
circle.draw();
cube.draw();
}
}
Output:
draw Circle
draw Cube
What is Partial Class?
Partial Class is a special class in C#. You can implement the functionality of a single class into multiple files, and these files should have the same name as the class, and all these files are combined into one class when the application is compiled.
In other words, you can split the implementation of a class into multiple files.
When to use Partial Class?
- When you have a large class, and you want to make your code lock good in UI code then use Partial Class.
- More than one developer can simultaneously work on the code for the same class.
Partial Class Key points
- It is declared using a partial keyword.
- The partial class allows dividing their properties, and methods into multiple source files, and at compile time the files are combined into a single class.
- Inheritance cannot be applied.
Example
public partial class Employee
{
public void DoWork()
{
Console.WriteLine("Working..");
}
}
public partial class Employee
{
public void GoToLunch()
{
Console.WriteLine("Eating..");
}
}
class Program
{
static void Main(string[] args)
{
var employee = new Employee();
employee.DoWork();
employee.GoToLunch();
}
}
OutPut:
Working…
Eating…
What is Static Class?
A static class is similar to a class that is both abstract and sealed. The difference between a static class and a non-static class is that a static class cannot be instantiated or inherited.
When to use Static Class?
- If your business logic of a method in the class doesn’t need to create an object, then use a static class by that you can use the methods in the class without creating an object.
Static Class Key points
- It is declared using the static keyword.
- Cannot create an object of the static class.
- Cannot be inherited.
- Inside a static class, only static members are allowed, which means everything inside the static class must be static.
- Methods in the class can be called using the class name without creating the instance.
Example
static class Person
{
// Static data members of Person
public static string Name = "Mohammed";
public static string Address = "Saudi Arabia";
// Static method of Person
public static void details()
{
Console.WriteLine("The details of Person is:");
}
}
public class Program
{
static public void Main()
{
// Calling static method of Person
Person.details();
// Accessing the static data members of Person
Console.WriteLine("Person Name: " + Person.Name);
Console.WriteLine("Person Address: " + Person.Address);
}
}
Output:
The details of Person is:
Person Name: Mohammed
Person Address: Saudi Arabia
What is Sealed Class?
A Sealed class is a class that cannot be inherited and used to restrict the properties. Once the class is Sealed then it can’t be inherited.
When to use Sealed Class?
- The main purpose of the sealed class is to withdraw the inheritance attribute from the user so that they cannot obtain a class from a sealed class.
- If a class isn't designed for an inheritance, then use the Sealed class.
Sealed Class Key points
- It is declared using the static keyword.
- Access modifiers are not applied to a sealed class.
- Cannot be inherited.
- To access the sealed members we must create an object of the class.
Example
sealed class SealedClass
{
public string name = "Mohammed";
public int Add(int x, int y)
{
return x + y;
}
}
public class Program
{
static public void Main()
{
SealedClass myObject = new SealedClass();
Console.WriteLine("name: " + myObject.name);
Console.WriteLine("sum of two number: " + myObject.Add(5, 2));
}
}
Output:
name: Mohammed
sum of two number: 7
References