In this post, we're gonna learn about strings in Python.
What's "string"?
- string is a data type like integers and other data types.
- string used to represent text.
- string is a sequence of characters that may contain spaces and numbers within it.
strings in Python
- Python does not have a character data type, a single character is a string with a length of 1.
- Python has a single string and multiline string.
Single line string in Python
The single string surrounded by either
- single quotation marks ' ',
- or double quotation marks " ".
Example
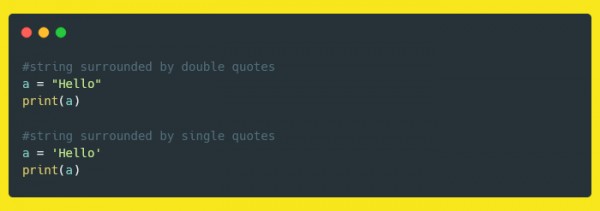
Multi-line string in python
The multi-line string in python surrounded by either
- three single quotation marks ''',
- or three double quotation marks """.
Example
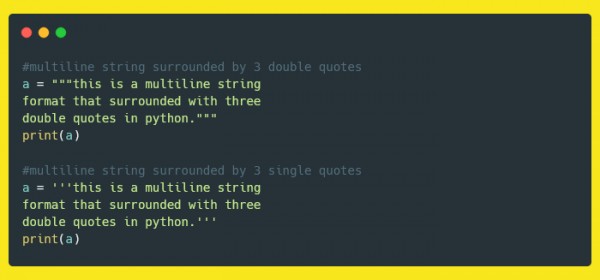
Python String Methods
In this section, we will list some of string methods with examples in Python.
capitalize()
It upper case the first letter of the given string.
Ex:
firstName="john"
capitalized_firstName=firstName.capitalize()
print(capitalized_firstName)
center()
It will center align the string using a specified character.
- It take two parameters string.center(length, character)
- length: required parameter > "the length of the returned string".
- character: optional parameter > "the default is space "the padding character".
Ex:
#defult align character
name="john"
centered_Name=name.center(8)
print(centered_Name)# john
#center align with charecter *
name="john"
centered_Name=name.center(8,'*')
print(centered_Name)#**john** the total length is 8
count()
Returns number of occurrences of a given substring.
- It takes three parameters string.count(substring, start, end)
- substring: required parameter.
- start: optional parameter that represents the starting index.
- end: optional parameter that represents the ending index.
Ex:
#defult count
name="john steven john"
substring = name.count("john")
print(substring)
# the output will be 2
#count with parameter
substring = name.count("john",4,15)
print(substring)
#the output will be 1
endswith()
Returns true or false depending on if the string ends with a given value or not.
- It takes a three-parameter string.endswith(value, start, end)
- value: Required parameter to check if the string ends with this value.
- start: optional parameter represents the starting index.
- end: optional parameter that represents the ending index.
Ex:
#defult endswith
name="john steven"
x = name.endswith("steven")
print(x)# the output will be true
#endswith with parameter
x = name.endswith("steven",2,8)
print(x) #the output will be false
startswith()
- The syntax is string.startswith(value, start, end)
- Returns true or false depending on if the string starts with a given value or not.
Ex:
name="john steven"
x = name.startswith("john")
print(x) # the output will be true
find()
- Returns the index of the first occurrence of the substring
- Returns -1 if not found.
- It takes a three-parameter string.find(substring, start, end)
- substring is required parameter "the string that will search for".
- start and end are optional parameters that represent the starting and ending index.
#defult find
name="john steven"
x = name.find("steven")
print(x)
# the output will be 5
#find with parameter
x = name.find("steven",2,8)
print(x)
#the output will be -1 "not found"
rfind()
- the Syntax is string.rfind(substring, start, end)
- Returns the index of the last occurrence of the substring.
- Returns -1 if not found.
rindex()
- It is same as rfind()
- the Syntax is string.rindex(substring, start, end)
- Returns the index of the last occurrence of the substring.
- Raises an exception if the value is not found.
islower()
- The syntax string.islower()
- Return True if all alphabets that exist in the string are lowercase.
- Return False if the string contains at least one uppercase.
isupper()
lower()
- The syntax is string.lower()
- Convert a given string to lowercase characters.
upper()
- The syntax is string.upper()
- Convert a given string to uppercase characters.
zfill()
- The syntax is string.zfill(length)
- adding '0' to the left of a given string until the string reach the specified length that the method takes as a parameter.
Ex:
In this example the string "Python" its length is 6 if the length specified to the length of 10 then the zfill(10) will return a string of length 10 so it will add '0000' to the left of the string to be '0000Python'.
If the length parameter is less than the original length, in this case, the zfill(length) will return the original string.
x="Python"
print(x.zfill(10)) # the output will be 0000Python
print(x.zfill(5)) # the output will be Python
title()
- The syntax is string.title()
- Convert the first letter of each word in the string to uppercase.
- Convert the letter after the non-alphabet letter to uppercase.
Ex:
x="welcome to python"
y="welcome 2a2a"
print(x.title()) # the output will be Welcome To Python
print(y.title()) #the output will be Welcome 2A2A
float()
- The syntax is float(value) value is option
- the value takes integer or float or string of decimal numbers
Ex:
Print(float(10)) //the output will be 10.0
Print(float(10.11)) //the output will be 10.11
Print(float(" 10.1\n")) //the output will be 10.1
isnumeric()
- The syntax is string.isnumeric()
- Return true if all string characters are numeric from 0 to 9 else it return false
Ex:
x="2563"
y="10k"
print(x.isnumeric()) #the output will be true
print(y.isnumeric()) #the output will be false
max()
- Return the max item from the given items
- It can take one or more of list, tuple, set, dictionary,etc
Ex:
x= [7, 2, 11, 5, 10, 6]
print(max(x)) #the output will be 11
replace()
- The syntax is replace(oldvalue,newvalue,[count]) #count is optional parameter
- It replaces a string part with another string "replace each occurrence of the string"
Ex:
x= " I love java"
print(x.replace("java",Python")) # the output will be I love Python
Conclusion
In this post, we explored the strings in Python and some of its important methods in detail.
See Also