In this post, we will learn about lists in Python ,how they are created, adding and removing items ,copy list,join lists and so on.
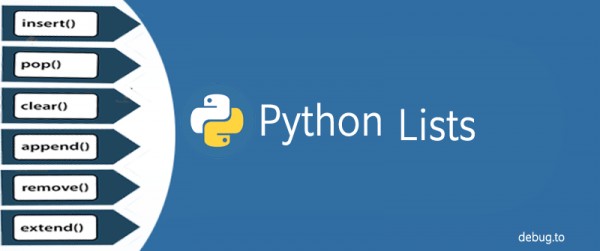
What's the list?
- The list is a type of container.
- The list is a data structure in Python that is a changeable and ordered sequence of elements.
- Lists allow duplicate members.
- In Python, lists are written with square brackets.
How to create lists in Python?
- The syntax is listName=[ ] .
- list is defined by having values between square brackets [ ] and the values are separated by a comma.
- list can have any numbers of items and these items may be in different types.
- List can contain other lists as items.
Ex:
#list with string elements
colorList=["Red", "Green", "White", "Black", "blue"]
#list with different data types
mylist=["red",1,1.2]
#list with nested lists
nestedList=[[1,2,3], "Red",[4,5]]
How to access list in Python?
- You can access the list item using the index operator.
- Nested lists can be accessed by using a nested index.
- Negative index means beginning from the end -1 index refers to the last item in the list, -2 the second item from the end, and so on.
- You can specify a range of indexes "start and the end" where the return value is a sub list from the original list, and this range can be positive or negative ranges.
Ex:
colorList=["Red", "Green", "White", "Black", "Blue"]
print(colorList[0]) # it return the first item Red
print(colorList[-1]) # it return the last item Blue
print(colorList[2:4])
# it return sub list from 2 (included) to 4 (excluded) ['White','Black']
print(colorList[-3:-1])
# it return sub list from -3 (included) to -1 (excluded) ['Blue','Black']
print(colorList[2:])
# it return sub list from 2 (included) to the end ['White','Black', 'Blue']
print(colorList[:2])
# it return sub list from the beginning to index 2 (excluded) ['Red','Green']
print(colorList[-2:])
# it return sub list from -2 (included) to the end ['Black', 'Blue']
print(colorList[:-2])
# it return sub list from the beginning to index -2 (excluded) ['Red','Green','White']
How to change list item value?
Change the list item value by using the item index.
Ex:
colorList=["Red", "Green", "White", "Black", "Blue"]
colorList[0]="yellow"
print(colorList)
# the output will be ['yellow', 'Green', 'White', 'Black', 'Blue']
Check if Exists
- check if a given item exists in the current list or not.
- It is performed by using in a keyword.
colorList=["Red", "Green", "White", "Black", "Blue"]
if "Red" in colorList:
print("Red is found")
Python list methods
append()
It adds item to the end of the list.
Ex:
colorList=["Red", "Green", "White", "Black", "Blue"]
colorList.append("orange")
print(colorList)
# the output will be ['Red','Green', 'White', 'Black','Blue','orange']
insert()
It add an item to a specific index.
Ex:
colorList=["Red", "Green", "White", "Black", "Blue"]
colorList.insert(1,"orange")
print(colorList)
# the output will be ['Red','orange','Green', 'White', 'Black','Blue']
remove()
It remove a specific item.
Ex:
colorList=["Red", "Green", "White", "Black", "Blue"]
colorList.remove("Red")
print(colorList)
# the output will be ['Green', 'White', 'Black','Blue']
extend()
It adds the content of one list to the end of the other list.
Ex:
in this example, we will add the content of the list which name is colorList2 to the end of colorList1
colorList1=["White", "Black", "Blue"]
colorList2=["Red", "Green"]
colorList1.extend("colorList2") print(colorList1)
# the output will be ['White', 'Black','Blue','Red','Green']
pop()
It removes items from a specific index. or the last index, if you do not specify an index.
Ex:
colorList=["Red", "Green", "White", "Black", "Blue"]
colorList.pop(1)
print(colorList)# the output will be ['Red', 'White', 'Black','Blue']
del keyword
- It removes item from a specific index.
- or it removes the list itself.
Ex:
colorList=["Red", "Green", "White", "Black", "Blue"]
del colorList[0]
print(colorList)# the output will be ['Green', 'White', 'Black','Blue']
del colorList # the list is no longer exist
copy()
It copies alist in another list
Ex:
colorList=["Red", "Green", "White", "Black", "Blue"]
copycolorList=colorList.copy()
print(copycolorList)#the output will be ['Red', 'Green', 'White', 'Black','Blue']
sum()
calculates the sum of all elements in the list.
sum used only for numeric values other wise it will raise a type error
Ex:
List=[1,2,3,4]
print(sum(List))
#the output will be 10
count()
used to calculate the numbers of occurrence for a specific element in the list.
Ex:
List=[1,2,1,3,4]
print(count(1))
#the output will be 2
len()
used to calculate the total number of elements in the list.
Ex:
List=[1,2,1,3,4]
print(len(List))
#the output will be 5
index()
used to return the first appearance of a specific element in the list.
Ex:
List=[1,2,1,2,3,4]
print(List.index(2))
#the output will be 1
min()
used to return the minimum of all elements in the list.
Ex:
List=[5,2,1,3,4]
print(min(List))
#the output will be 1
max()
used to return the maximum of all elements in the list.
Ex:
List=[5,2,1,3,4]
print(max(List))
#the output will be 5
Conclusion
In this post, we have explored python lists and how we can use it
See Also