In this post, we're gonna learn how to handle Exception in Python.
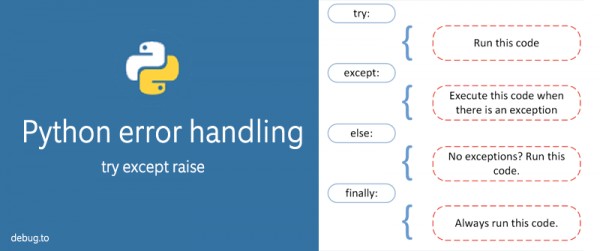
Before we getting started, let's first know more about errors and exceptions
Errors
Error is a problem within the program that can't be fixed by the program.
We can categorize the errors in python in two errors:-
- Compile Errors: that kind of errors occur when you ask Python to run your application in the time when your code compiled to machine code, the program can not be run before you fix the error, the most common type of this error is "Syntax error".
Example
in this example, it will raise syntax error because you don't end the if statement with the colon
a=1
b=2
if b>a
print("b is greater than a")
Example
in this example, it will raise runtime error when you try to divide by zero
a=1
b=0
z=a/b
print(z)
How to fix the errors?
We used to define the error by its location, type, and details as the first line contain the location of the error then you can use your logic to solve it.
Python Error handling
- Handling the error means when the error occurs the program will normally stop and throw an error message.
- Python has many built-in exceptions that force your program to throw an error message when an error occurs.
- We can handle the exceptions using try
Try-Except statement
Use: Try statement is using to handle the exception the code that can cause a problem we will put it in the try block and the catching error "the error message" we will put it in the except block.
Syntax:
try:
# the code that can cause a problem
except:
#the error message that we will inform the user by it
Example:
In this example, we try to print the variable x but it didn't find it in the program so the program will crash and raise an error, in order to control the error message we will raise the error in the except block
try:
print(x)
except:
print("An exception occurred x not found")
Try-Except-else statement
Use: It is the same as try-except besides else block that will execute if no exception is found.
Syntax:
try:
# the code that can cause a problem
except:
#the error message that we will inform the user by it
else:
#this block will execute when no exception is found
Example
in this example, we will ask the user to enter a number,
if the number is not int we will handle an exception,
else if the number is int we will check if the number is less than or greater than 2 and print a message to the user.
try:
x=int(input('Enter your number '))
except:
print ('You have entered an invalid value.')
else:
if x < 2:
print('You are not allowed to enter number less than 2')
else:
print(' you entered valid number that greater than 2.')
Finally
use: It is running if the try statement raised an error or not.
Syntax:
try:
# the code that can cause a problem
except:
#the error message that we will inform the user by it
finally:
#the code that always be executed if the try statement raise an error or not
Handling a specific type of exceptions
As you can define as many exceptions you want, you also can catch a specific type of exception.
example:
in this example, we can catch NameError exception and you can use exceptions as you want.
try:
print(x)
except NameError:
print("Variable x is not defined in your code")
except:
print("there is an error but not a name error ")# you can write your own error message.
Raise exception
use: you can raise an exception depending on a kind of error you defined "predefined condition ", and you can define what kind of error you can raise.
syntax:
raise exception
Example:
x = -1
if x < 0:
raise Exception("Sorry, not a Positive number")
example of type error exception
x = "Python"
if not type(x) is int:
raise TypeError("Only integers values of x are allowed")
Conclusion
In this post, we have learned how to handle exceptions using
- try-except
- try-except-else
- finally
- raise exception
- raise specific kind of exceptions
in Python programming language.
See Also