What's the Liskov Substitution Principle?
In simple terms, the Liskov Substitution Principle (LSP) posits that if a subclass 'B' extends or inherits from a base class 'A,' then instances of class 'B' should seamlessly substitute instances of class 'A' without causing any bug or exceptions.
Alternative definition : The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program
Example Description:
I aim to develop an application designed for the efficient storage of files for both management and employees within the government sector. The specific requirement involves storing management files in a SharePoint list, while files for general employees will be stored in an SQL database.
How to implement this case using SOLID focus on LSP ?
To implement this case without using LSP , please follow below steps :
- It's Un-PREFERRED soluation
- In below code define abstract class " Files " that contain 2 abstract function that override in any class inherate from this class .
- Create classes " GeneralEmployees - Managerial " that inherate from Files
- Call 2 function in program.cs
public abstract class Files
{
public abstract void SaveFilesInSharePointList();
public abstract void SaveFilesInSQLDB();
}
public class GerneralEmployees : Files
{
public override void SaveFilesInSharePointList()
{
throw new NotImplementedException();
}
public override void SaveFilesInSQLDB()
{
// TODO Logic here
Console.WriteLine("Saved files from `GerneralEmployees` into SQL DB ");
}
}
public class Managerial : Files
{
public override void SaveFilesInSharePointList()
{
Console.WriteLine("Saved files from `Managerial` into SP List ");
}
public override void SaveFilesInSQLDB()
{
throw new NotImplementedException();
}
}
using LiskovSubstitutionPrinciple.Services;
Files gerneralEmployees = new GerneralEmployees();
DisplayMessage(gerneralEmployees);
Managerial managerial = new Managerial();
DisplayMessage(managerial);
static void DisplayMessage(Files operation)
{
operation.SaveFilesInSQLDB();
operation.SaveFilesInSharePointList();
}
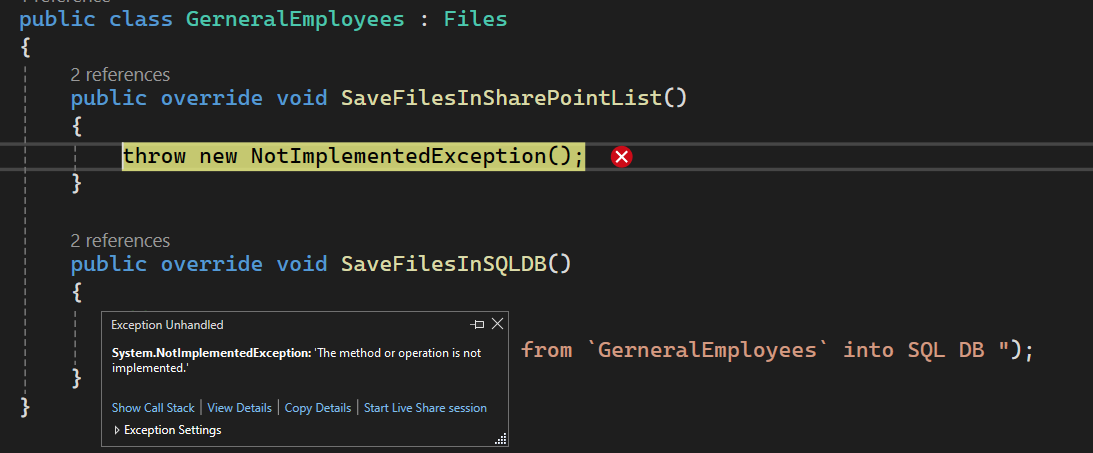
Some notes for above example , when created Files class and GerneralEmployees inherate from Files it's not supposably to implement SaveFilesInSharePointList and same case in GerneralEmployees
that made exception when call method i don't want in this case ,, which made this case violates the LSP principle
- It's PREFERRED soluation that apply LSP
- I created abstract class with name " FilesOperations "
- Created 2 classes with name " GeneralManager and Managerial " that override function " SaveFiles " and every class implement it's logic .
- Execute objects in Program.cs and run successfully without any exception after applying LS principle .
public abstract class FilesOperation
{
public abstract void SaveFiles();
}
public class GerneralEmployees : FilesOperation
{
public override void SaveFiles()
{
Console.WriteLine("Saved files from `GerneralEmployees` into SQL DB ");
}
}
public class Managerial : FilesOperation
{
public override void SaveFiles()
{
Console.WriteLine("Saved files from `Managerial` into SP List ");
}
}
using LiskovSubstitutionPrinciple.Services;
FilesOperation gerneralEmployees = new GerneralEmployees();
DisplayMessage(gerneralEmployees);
FilesOperation managerial = new Managerial();
DisplayMessage(managerial);
static void DisplayMessage(FilesOperation operation)
{
operation.SaveFiles();
}
Result:
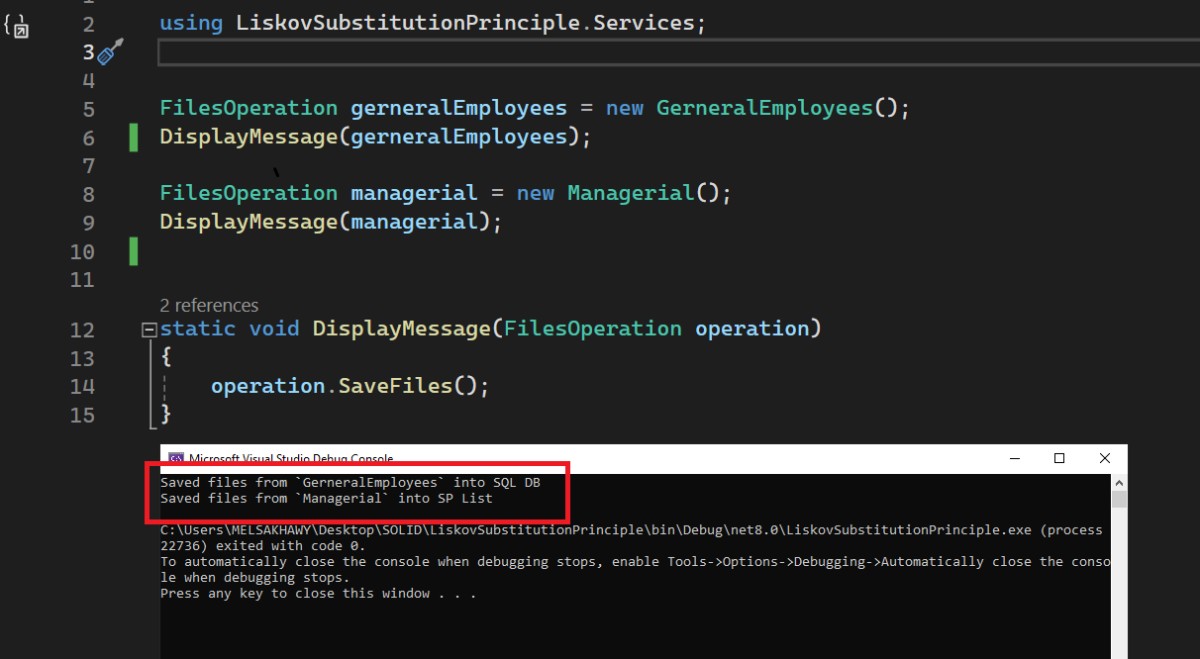